Python Programming
1 Basic Python Knowledge
1.1 Strings
1.1.1 String Representation
In Python, all objects produce two string representations:
The str is legible to humans.
The repr is legible to Python interpreter.
repr: Provides a string representation that can be used to recreate the object. It's designed for debugging and introspection. It aims for a more precise and detailed description, often including the object's type.
Examples:
class MyClass: def __init__(self, value): self.value = value def __repr__(self): return f"MyClass({self.value})" obj = MyClass(10) print(repr(obj)) # Output: MyClass(10)repr: Provides a human- readable string representation of the object. It's designed to be easily understood by humans.
Examples:
class MyClass: def __init__(self, value): self.value = value def __str__(self): return f"MyClass with value: {self.value}" obj = MyClass(10) print(str(obj)) # Output: MyClass with value: 10
1.1.2 Strings in Python
For more information on strings, please visit strings in Java.
Examples in Python:
1.2 Lists
Examples:
1.3 Tuple
Examples:
1.4 Set
Set cannot contain unhashable type aka mutable type.
Hashable type: int, float, string, tuple, bool.
Unhashable type: list, set, dict.
1.5 Dictionary
1.6 Bytes
ASCII: 1 bytes, 8 bits.
ANSI: A standard. 2 bytes, 16 bits.
Mainland China: GB2312 => GBK (Windows).
Taiwan, China: Big5.
Japan: JIS.
Unicode:
UCS-2: 2 bytes, 16 bits.
UCS-4: 4 bytes, 32 bits .
UTF: All the same as Unicode, except that the length is changeable.
English: 1 byte, 8 bits .
Some of European languages: 2 bytes, 16 bits.
Chinese: 3 bytes, 24 bits.
UTF-16: Shortest length is 16 bits.
1.7 Logical Operators
Priority:
() => not => and => or
2 Higher-Order Function
Higher-order function: A function that takes a function as an argument value or returns a function as a return value.
2.1 Higher-Order Function (Functions as Arguments)
Examples:
Apply a User-Defined Function
Create a new frame.
Bind formal parameters (f & x) to arguments.
Execute the body: return f(f(x)).
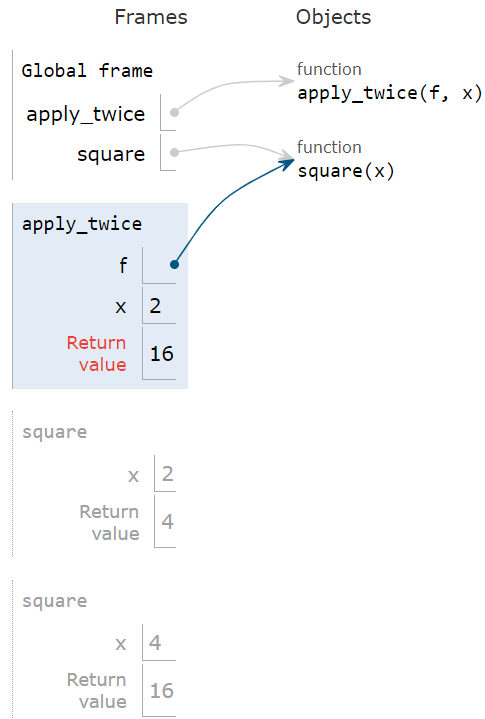
2.2 Nested Definitions (Functions as Returned Values)
Propositions:
Every user-defined function has a parent frame (often global).
The parent of a function is the frame in which it was defined.
Every local frame has a parent frame (often global).
The parent of a frame is the parent of function called.
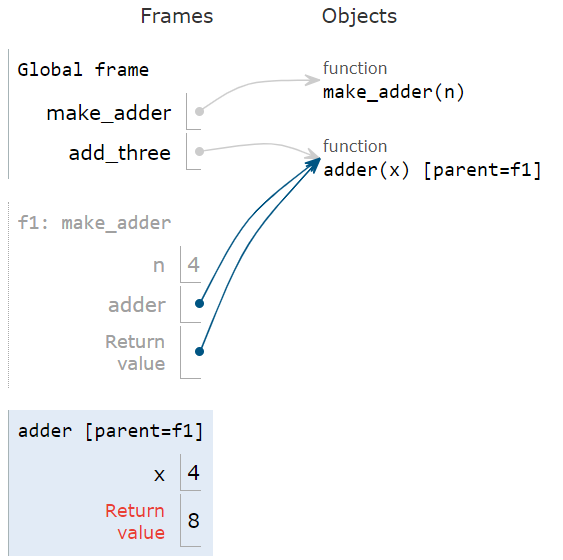
2.3 Lambda Expressions
Important notes:
No "return" keyword!
Lambda expressions are not common in Python, but important in general.
Lambda expressions in Python cannot contain statements at all!
2.4 Currying
Examples:
3 Recursion
Recursive Function: A function is called recursive if the body of that function calls itself, either directly or indirectly.
3.1 Self-Reference: Return by its own name
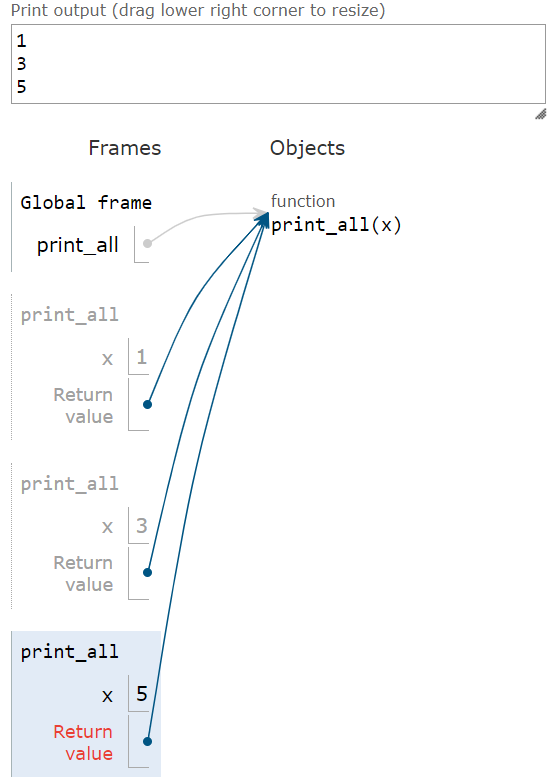
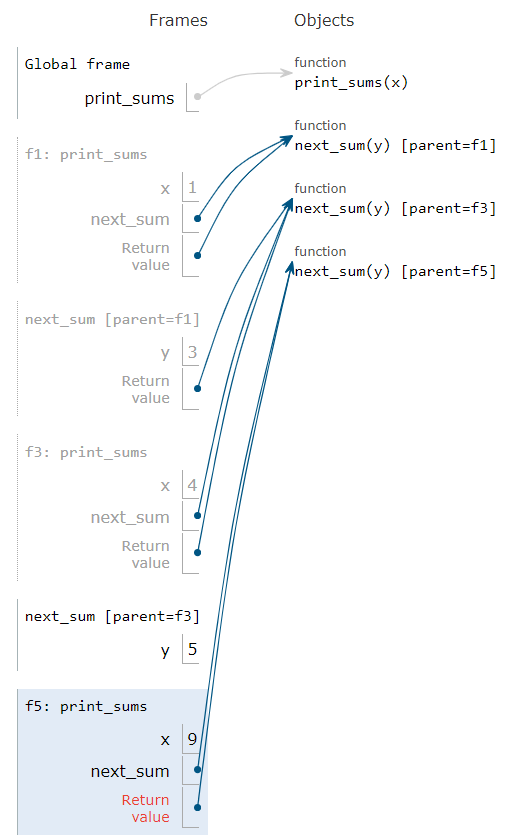
3.2 Recursion & Environment Diagrams
Example 1:
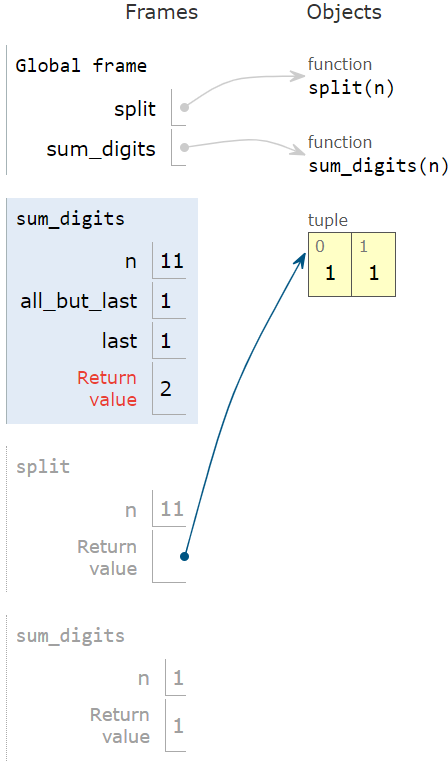
Example 2:
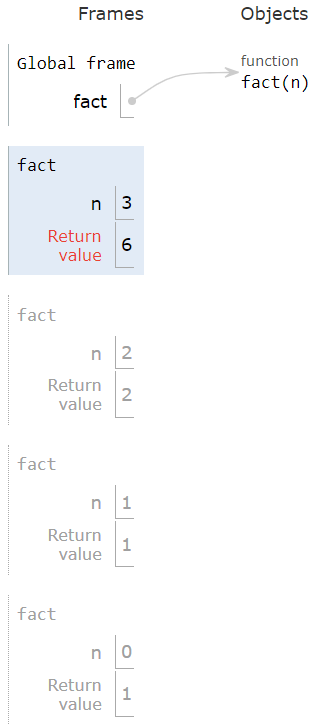
3.3 Iteration & Recursion
Iteration | Recursion | |
---|---|---|
Sample Implementation | Using while:
def fact_iter(n):
total, k = 1, 1
while k <= n:
total, k = total * k, k + 1
return total
| Using recursion:
def fact(n):
if n == 0:
return 1
else:
return n * fact(n - 1)
|
Math | ||
Conversion (to another) | More formulaic: The state of an iteration can be passed as arguments. | Can be tricky: Find out what state must be maintained by the iterative function. |
3.4 Mutual Recursion
Luhn Algorithm - Used to verify credit card numbers.
From the rightmost digit, which is the check digit, moving left, double the value of every second digit; if product of this doubling operation is greater than 9 (e.g.,
), then sum the digits of the products (e.g., 10: , 14: ). Take the sum of all the digits. The Luhn sum of a valid credit card number is a multiple of 10.
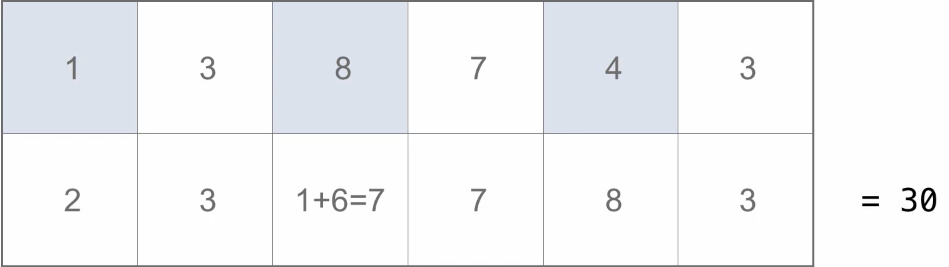
4 Iterators & Generators
4.1 Iterators
Definitions:
Iterable: An object capable of returning its members one at a time.
Iterator: An object that progressively provides access to each item of a collection, in order.
Types of iterables:
Operations on iterators:
iter (iterable): Return an iterator over the elements of an iterable value.
next (iterable): Return the next element in an iterator.
Example usage:
4.2 Iterables
Lists: [1, 2, 3, 4]
Tuples: (10, 20, 30)
Strings: "Hello"
Dictionaries: {"name": "Alice", "age": 30} (iterates over keys by default)
Sets: {1, 2, 3}
Ranges: range(1, 5)
File Objects: Used for reading data from files line by line.
Special case: Dictionaries
The order of items of a dictionary is the order in which they were added (Python 3.6+).
Historically, items appeared in an arbitrary order (Python 3.5 and earlier).
Example usage:
4.3 Generators
Definitions:
Generator: A function that yields value instead of returning them.
Generator: An iterator created automatically by calling a generator function.
Example usage:
Generators can yield form iterators:
A yield from statement yields all values from an iterator or iterable (Python 3.3).
Example 1:
Example 2:
4.4 Built-In Iterator Functions
Many built-in Python sequence operations return iterators that compute results lazily.
To view the contents of an iterator, place the resulting elements into a container.
list (iterable): Create a list containing all x in iterable.
tuple (iterable): Create a tuple containing all x in iterable.
sorted (iterable): Create a sorted list containing x in iterable.
4.4.1 Map
map (func, iterable): Iterate over func(x) for x in iterable.
Example usage:
4.4.2 Filter
filter (func, iterable): Iterate over x in iterable if func(x).
Example usage:
4.4.3 zip
zip (first_iter, second_iter, ...): Iterate over co-indexed (x, y) pairs.
Example usage:
Example 1:
Example 2:
4.4.4 reversed
reversed (sequence): Iterate over x in a sequence in reverse order.
Example usage:
4.4.5 range iterator
Example usage:
5 Efficiency
5.1 Order of Growth
For more information on order of growth, please refer to Data Structures and Algorithms 1.
5.2 Space
At any moment there is a set of active environments. Values and frames in active environments consume memory.
Memory that is used for other values and frames can be recycled.
Active Environments:
Environments for any function calls currently being evaluated => call the function but hasn't returned yet.
Parent environments of functions named in active environments => define a function in another function, so the function defined is not in the global frame, the parent frame is needed.
6 Object-Oriented Programming
6.1 Object-Oriented Programming in Python
For more information about the details of Object-Oriented Programming, refer to Object-Oriented Programming in C++.
When a class is called:
A new instance of that class (aka an object) is created.
The _init_ method of the class is called with the new object as its first argument (named self), along with any additional arguments provided in the call expression.
An object's attributes can be accessed and modified using dot expressions.
Every object that is an instance of a class has a unique identity.
All invoked methods have access to the object via the self parameter, and so they can all access and manipulate the object's attributes.
6.2 Inheritance
For more information about inheritance, please visit Object-Oriented Programming in C++.
6.3 Special Method Functions
Certain names are special because they have built-in behavior.
These names always start and end with two underscores.
__init__ Method invoked automatically when an object is constructed
__repr__ Method invoked to display an object as a Python expression
__add__ Method invoked to add one object to another
__bool__ Method invoked to convert an object to True or False.
__float__ Method invoked to convert an object to a float (real number).
7 Scheme
7.1 Scheme Fundamentals
Scheme programs consist of expressions, which can be:
Primitive expressions: 2, 3.3, true, +, quotient...
Combinations: (quotient 10 2), (not true)...
Numbers are self-evaluating; symbols are bound to values.
Call expressions include an operator and 0 or more operands in parenthesis.
Example:
Special forms:
Special forms: A combination taht is not a call expression is a special form:
If statement : (if <predicate> <consequent> <alternative >)
And and or: (and <e 1 > ... <e n >), (or <e 1 > ... <e n >)
Binding symbols: (define < symbol> <expression>)
New procedures: (define < symbol> <formal parameters>) <body>
Lambda Expressions: (lambda (<formal-parameters>) <body>
Examples:
Cond & Begin:
Cond
Begin
Let:
7.2 Scheme Lists
cons: Two-argument procedure that creates a linked list.
car: Procedure that returns the first element of the list.
cdr: Procedure that returns the rest of a list.
nil: The empty list.
Symbolic programming:
Built-In List Processing Procedures
(append s t): list the elements of s and t; append can be called on more than 2 lists.
(map f s): call a procedure f on each element of a list s and list the results.
(filter f s): call a procedure f on each element of a list s and list the elements for which a true value is the result.
(apply f s): call a procedure f with the elements of a list as its arguments.