Part Ⅲ
16 Minimum Spanning Trees
16.1 Introduction to MSTs
Spanning tree: A spanning tree is a subgraph
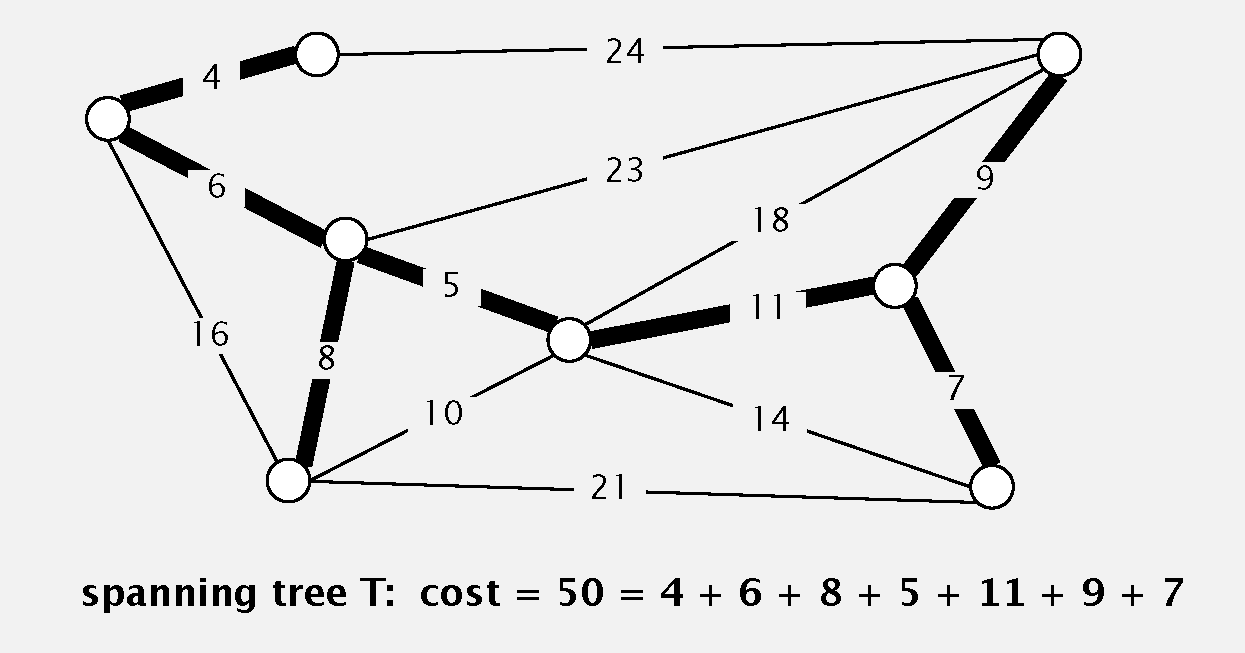
Application:
Dithering
Cluster analysis
Max bottleneck paths
Real-time face verification
LDPC codes for error correction
Image registration with Renyi entropy
Find road networks in satellite and aerial imagery
Reducing data storage in sequencing amino acids in a protein
Model locality of particle interactions in turbulent fluid flows
Autoconfig protocol for Ethernet bridging to avoid cycles in a network
Approximation algorithms for NP-hard problems (e.g., TSP, Steiner tree)
Network design (communication, electrical, hydraulic, computer, road)
16.2 Greedy Algorithm
Definitions
Cut: A cut in a graph is a partition of its vertices into two (nonempty) sets.
Crossing edge: A crossing edge connects a vertex in one set with a vertex in the other.
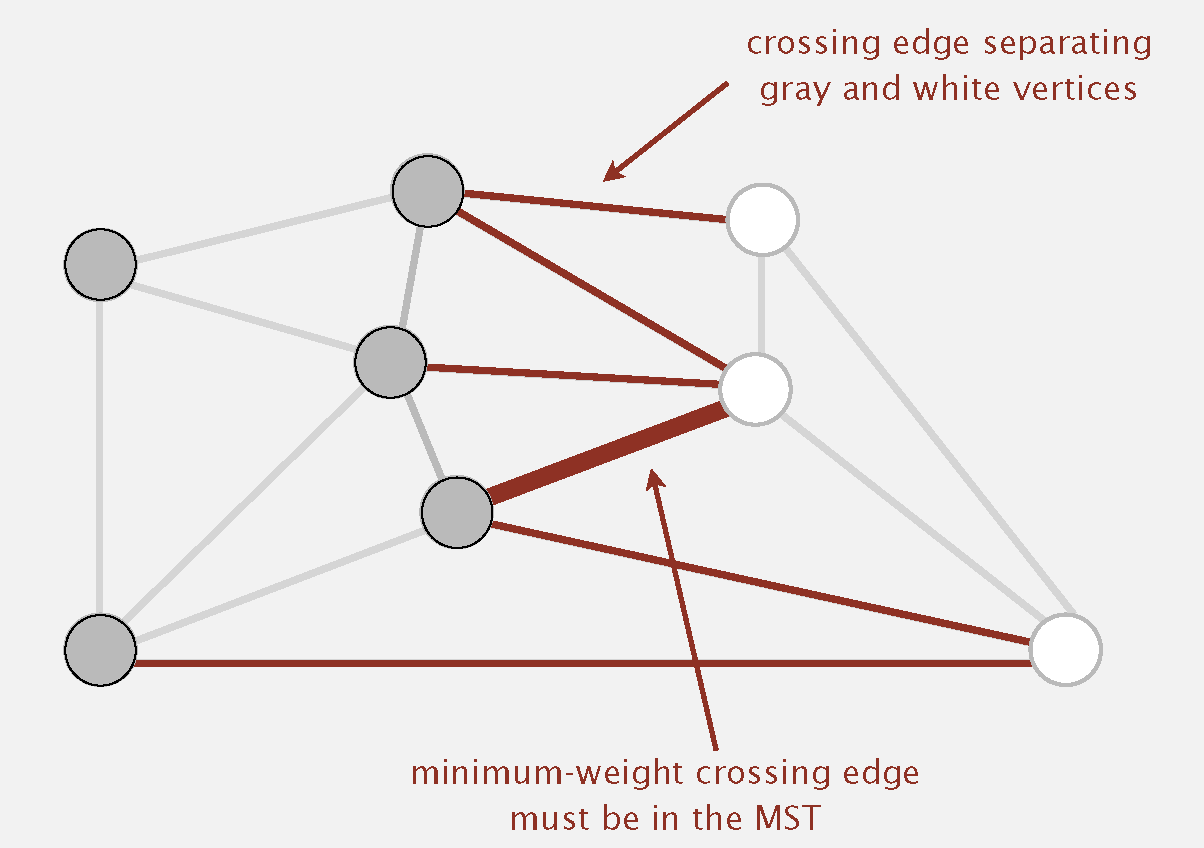
Greedy Algorithm for MST
Start with all edges colored gray.
Find cut with no black crossing edges; color its min-weight edge black.
Repeat until
edges are colored black.
Correctness Proof
Given any cut, the crossing edge of min weight is in MST.
Proof
Suppose min-weight crossing edge
is not in the MST. Adding
to the MST creates a cycle. Some other edge
in cycle must be a crossing edge. Removing
and adding is also a spanning edge. Since weight of
is less than the weight of , that spanning tree is lower height. Contradiction.
The greedy algorithm computes the MST.
Proof
Any edge colored black is in the MST (via cut property).
Fewer than
black edges => cut with no black crossing edges (consider cut whose vertices are one connected component).
16.3 Edge-weighted Graph Implementation
Edge
Edge-weighted Graph
16.4 Kruskal's Algorithm
Kruskal's Algorithm
Consider edges in ascending order of weight.
Add next edge to tree
unless doing so would create a cycle.
Union-Find for Cycle Challenge
Maintain a set for each connected component in
If
and are in same set, then adding would create a cycle. To add
to , merge sets containing and .
Correctness Proof
Kruskal's Algorithm is a special case of the greedy MST algorithm.
Suppose Kruskal's algorithm colors the edge
black. Cut = set of vertices connected to
in tree . No crossing edge is black.
No crossing edge has lower weight.
Property: Kruskal's algorithm computes MST in time proportional to
Proof
Operation | Frequency | Time per op |
---|---|---|
Build pq | ||
Delete-min | ||
Build pq | ||
Connected |
16.5 Prim's Algorithm
Prim's Algorithm
Start with vertex
and greedily grow tree Add to
the min weight edge with exactly one endpoint in . Repeat until
edges.
Correctness Proof
Prim's Algorithm is a special case of the greedy MST algorithm.
Suppose edge e = min weight edge connecting a vertex on the tree to a vertex not on the tree.
Cut = set of vertices connected on tree.
No crossing edge is black.
No crossing edge has lower weight.
16.5.1 Lazy Implementation
Lazy Implementation
Maintain a PQ of edges with (at least) one endpoint in T.
Key = edge; priority = weight of edge.
Delete-min to determine next edge
to add to . Disregard if both endpoints
and are in . Otherwise, let
be the vertex not in . Add to PQ any edge incident to
(assuming other endpoint not in T) Add
to and mark .
Property: Lazy Prim's algorithm computes the MST in time proportional to
Operation | Frequency | Binary Heap |
---|---|---|
Delete min | ||
Insert |
16.5.2 Eager Implementation
Property
Running time depends on PQ implementation:
PQ Implementation | Insert | Delete-Min | Decrease-Key | Total |
---|---|---|---|---|
Array | ||||
Binary Heap | ||||
d-way Heap (Johnson 1975) | ||||
Fibonacci Heap (Fredman-Tarjan 1984) |
*: amortized
Bottom Line
Array implementation optimal for dense graph.
Binary heap much faster for sparse graphs.
4-way heap worth the trouble in performance-critical situations.
Fibonacci heap best in theory, but not worth implementing.
16.6 MST Context
16.6.1 Euclidean MST
Euclidean MST: Given
Methods: Exploit geometry and do it in
16.6.2 Single Link Clustering
Definitions
k-clustering: Divide a set of objects calssify into
coherent groups. Distance Function: Numeric value specifying "closeness" of two objects.
Single link: Distance between two clusters equals the distance between the two closest objects (one in each cluster).
Single-link clustering: Given an integer k, find a k-clustering that maximizes the distance between two closest clusters.
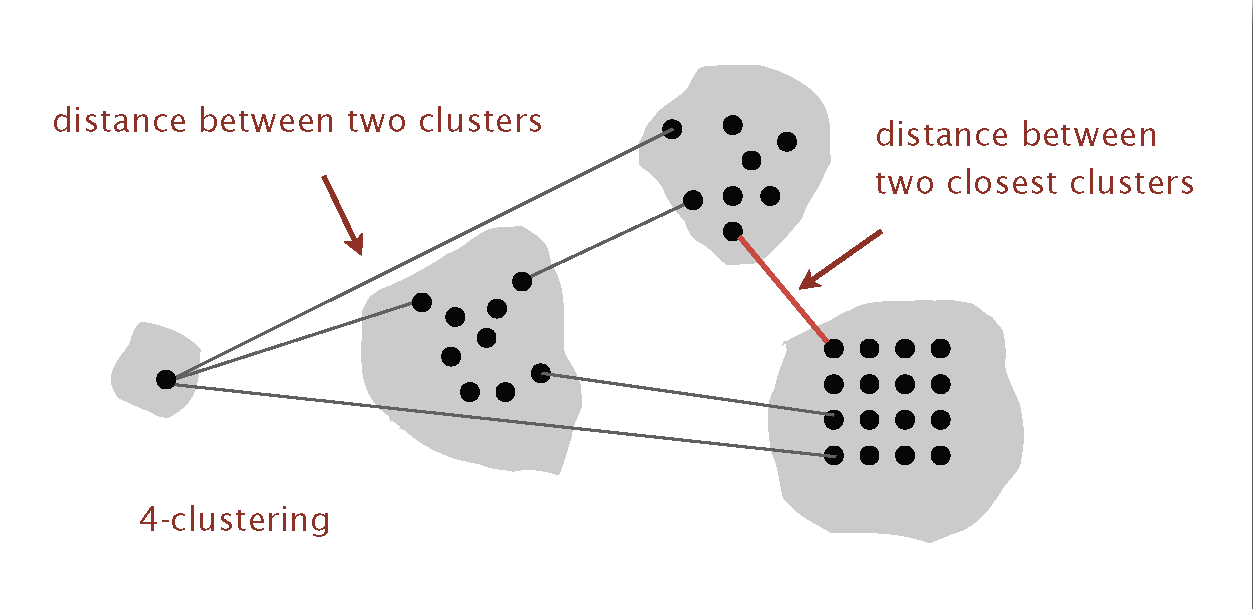
"Well-known" algorithm in science literature for single-link clustering:
Form
clusters of one object each. Find the closest pair of objects such that each object is in a different cluster, and merge the two clusters.
Repeat until there are exactly
clusters.
Applications
Routing in mobile ad hoc networks.
Document categorization for web search.
Similarity searching in medical image databases.
Skycat: cluster
sky objects into stars, quasars, galaxies.
16.7 Important Questions
Q: Bottleneck minimum spanning tree: Given a connected edge-weighted graph, design an efficient algorithm to find a minimum bottleneck spanning tree. The bottleneck capacity of a spanning tree is the weights of its largest edge. A minimum bottleneck spanning tree is a spanning tree of minimum bottleneck capacity.
A: Prove that an MST is a minimum bottleneck spanning tree.
Q: Is an edge in a MST: Given an edge-weighted graph
and an edge , design a linear-time algorithm to determine whether appears in some MST of . Note: Since your algorithm must take linear time in the worst case, you cannot afford to compute the MST itself.
A: Consider the subgraph
of containing only those edges whose weight is strictly less than that of . Q: Minimum-weight feedback edge set: A feedback edge set of a graph is a subset of edges that contains at least one edge from every cycle in the graph. If the edges of a feedback edge set are removed, the resulting graph is acyclic. Given an edge-weighted graph, design an efficient algorithm to find a feedback edge set of minimum weight. Assume the edge weights are positive.
A: Complement of an MST.
17 Shortest Paths
17.1 Shortest Paths APIs
Goal: Given an edge-weighted digraph, find the shortest path from
Navigation
PERT/CPM
Map routing
Seam carving
Robot navigation
Texture mapping
Typesetting in TeX
Urban traffic planning
Optimal pipelining of VLSI chip
Telemarketer operator scheduling
Routing of telecommunications messages
Network routing protocols (OSPF, BGP, RIP)
Exploiting arbitrage opportunities in currency exchange
Optimal truck routing through given traffic congestion pattern
Directed Edge
Edge-Weighted Digraph
17.2 Shortest Path Properties
Cost of undirected shortest paths:
Proof: Undirected shortest paths (with nonnegative weights) reduces to directed shortest path.
cost of shortest paths in digraph cost of reduction
Edge Relaxation
distTo[v]
is length of shortest known path fromto . distTo[w]
is length of shortest known path fromto . edgeTo[w]
is last edge on shortest known path fromto . If
gives shorter path to through , update both distTo[w]
andedgeTo[w]
.
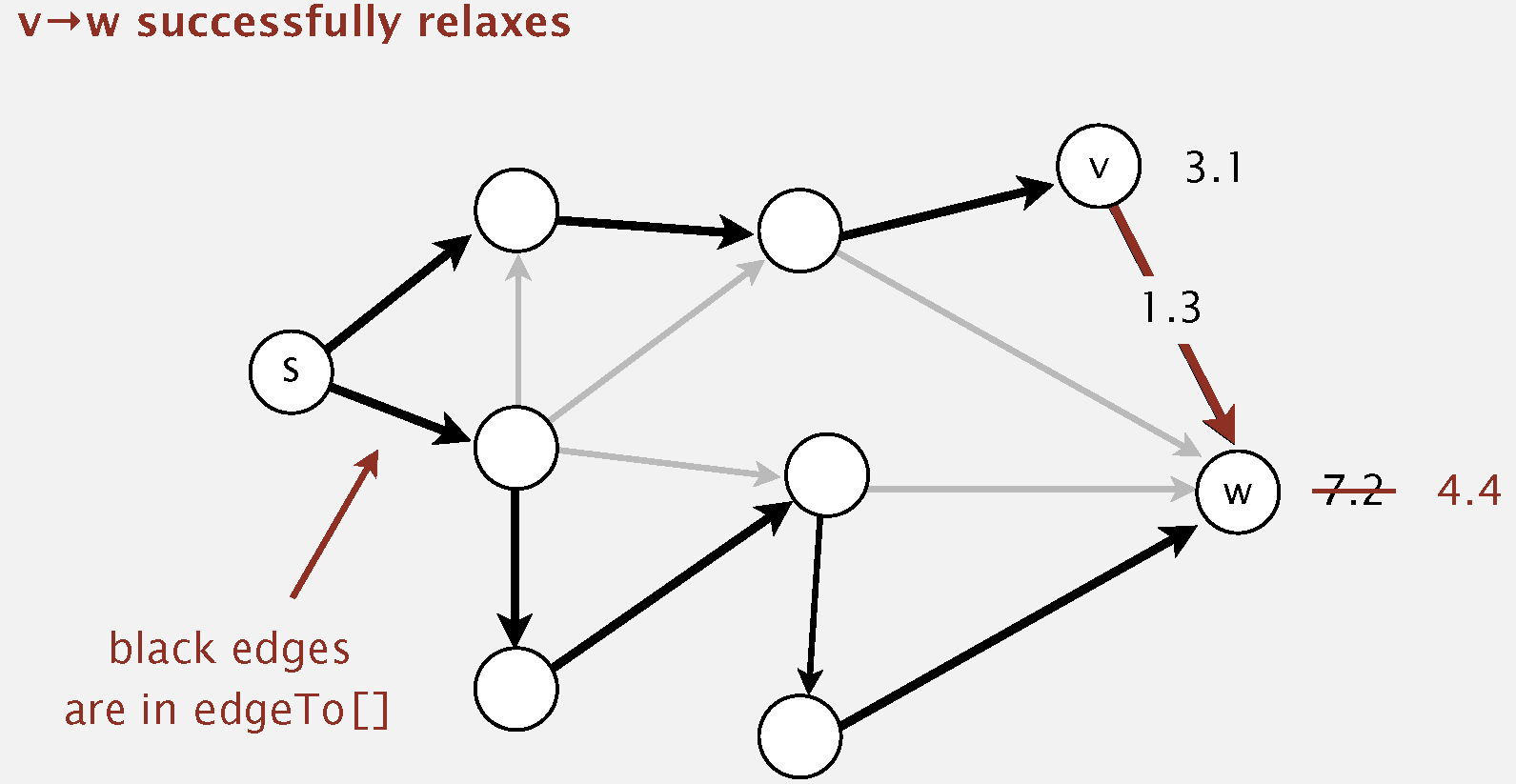
Correctness Proof: Shortest-paths optimality conditions
Let
Then distTo[]
are the shortest path distances from
distTo[s] = 0.
For each vertex v, distTo[v] is the length of some path from
to . For each edge
, distTo[w] <= distTo[v] + e.weight().
Proof
Suppose that
is a shortest path from to . Then,
= edge on shortest path from to . Add inequalities; simplify; and substitute
is the weight of shortest path from to . Thus,
distTo[w]
is the weight of shortest path to.
Different Implementations
Dijkstra's algorithm (nonnegative weights).
Topological sort algorithm (no directed cycles).
Bellman-Ford algorithm (no negative cycles).
17.3 Dijkstra's Algorithm
Dijkstra's Algorithm
Consider vertices in increasing order of distance from s.
(non-tree vertex with the lowest
distTo[]
value)Add vertex to tree and relaw all edges pointing from that vertex.
Correctness Proof: Dijkstra's algorithm computes a SPT in any edge-weighted digraph with nonnegative weights.
Each edge
is relaxed exactly once (when v is relaxed), leaving . Inequality holds until algorithm terminates because:
distTo[w]
cannot increase =>distTo[]
values are monotone decreasing.distTo[v]
will not change => we choose lowestdistTo[]
value at each step and edge weights are nonnegative)
Thus, upon termination, shortest-paths optimality conditions hold.
Prim’s algorithm is essentially the same algorithm as Dijkstra's algorithm
Both are in a family of algorithms that compute a graph's spanning tree.
Prim's: Closest vertex to the tree (via an undirected edge).
Dijkstra's: Closest vertex to the source (via a directed path).
Property
Running time depends on PQ implementation:
PQ Implementation | Insert | Delete-Min | Decrease-Key | Total |
---|---|---|---|---|
Array | ||||
Binary Heap | ||||
d-way Heap (Johnson 1975) | ||||
Fibonacci Heap (Fredman-Tarjan 1984) |
*: amortized
Bottom Line
Array implementation optimal for dense graph.
Binary heap much faster for sparse graphs.
4-way heap worth the trouble in performance-critical situations.
Fibonacci heap best in theory, but not worth implementing.
17.4 Edge-Weighted DAGs
Topological Sort Algorithm for Shortest Path
Consider all vertices in topological order.
Relax all edges pointing from that vertex.
Property: Topological sort algorithm computes SPT in any edgeweighted DAG in time proportional to
Each edge
is relaxed exactly once (when v is relaxed), leaving . Inequality holds until algorithm terminates because:
distTo[w]
cannot increase =>distTo[]
values are monotone decreasing.distTo[v]
will not change => we choose lowestdistTo[]
value at each step and edge weights are nonnegative)
Thus, upon termination, shortest-paths optimality conditions hold.
Longest paths in edge-weighted DAGs:
Formulate as a shortest paths problem in edge-weighted DAGs.
Negate all weights.
Find shortest paths.
Negate weights in result.
Application Ⅰ - Content-Aware Resizing
Seam Carving: Resize an image without distortion for display on cell phones and web browsers.
Grid DAG: vertex = pixel; edge = from pixel to 3 downward neighbors.
Weight of pixel = energy function of 8 neighboring pixels.
Seam = shortest path (sum of vertex weights) from top to bottom.
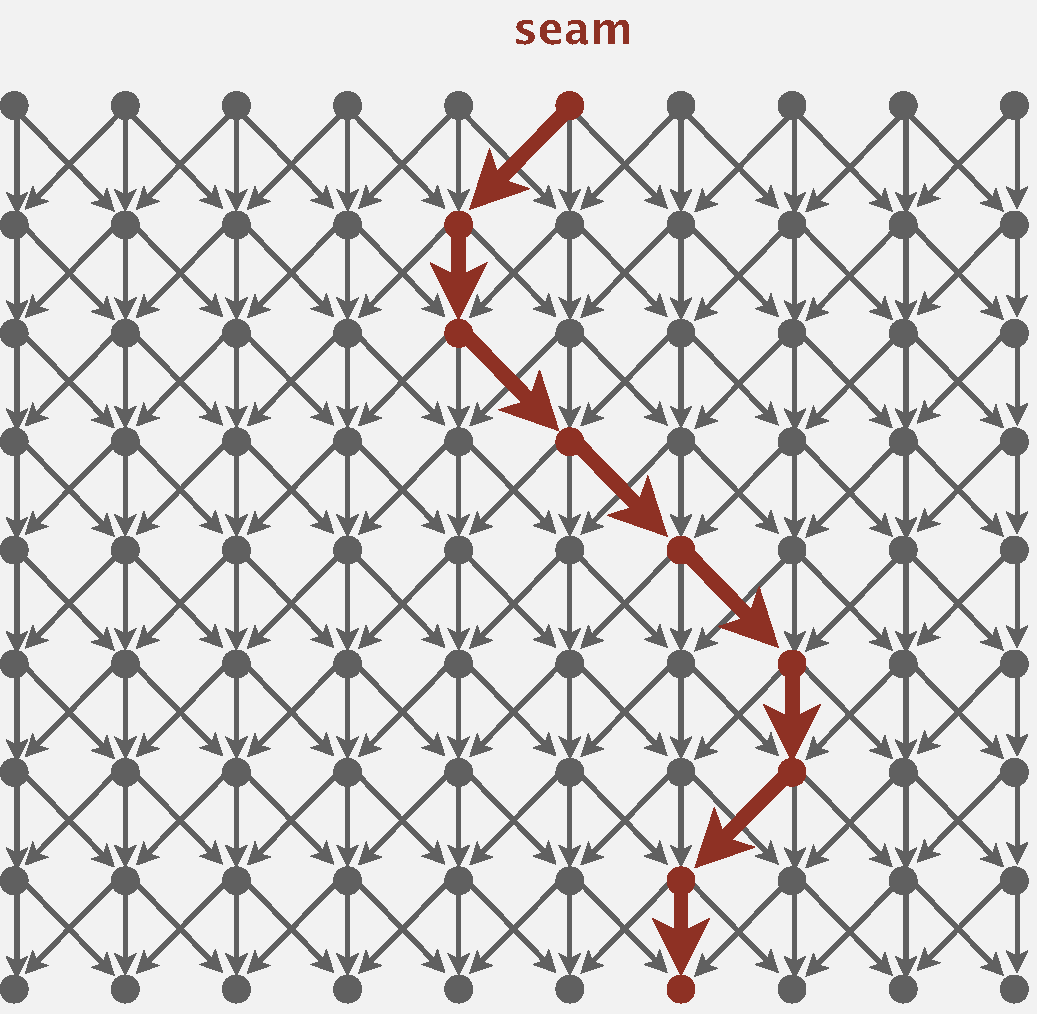
Application Ⅱ - Parallel Job Scheduling
Parallel Job Scheduling: Given a set of jobs with durations and precedence constraints, schedule the jobs (by finding a start time for each) so as to achieve the minimum completion time, while respecting the constraints.
To solve a parallel job-scheduling problem, create edge-weighted DAG, use longest path from the source to schedule each job:
Source and sink vertices.
Two vertices (begin and end) for each job.
Three edges for each job.
begin to end (weighted by duration)
source to begin (0 weight)
end to sink (0 weight)
One edge for each precedence constraint (0 weight).
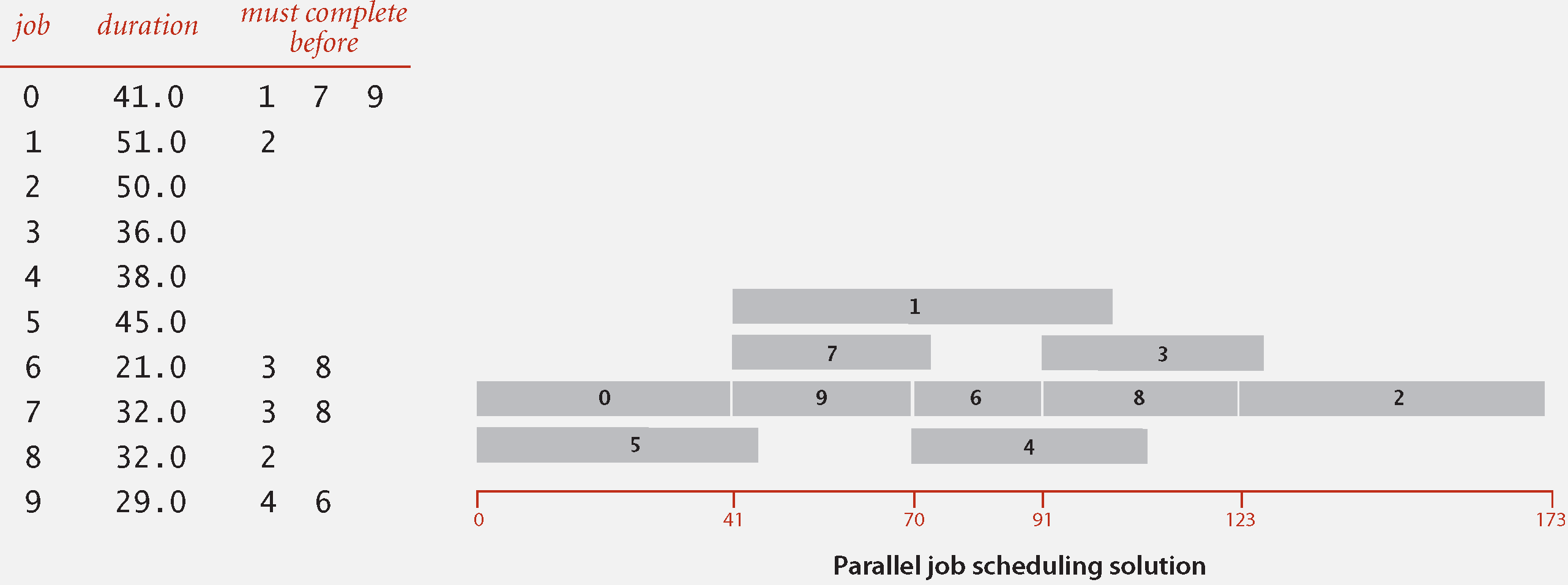
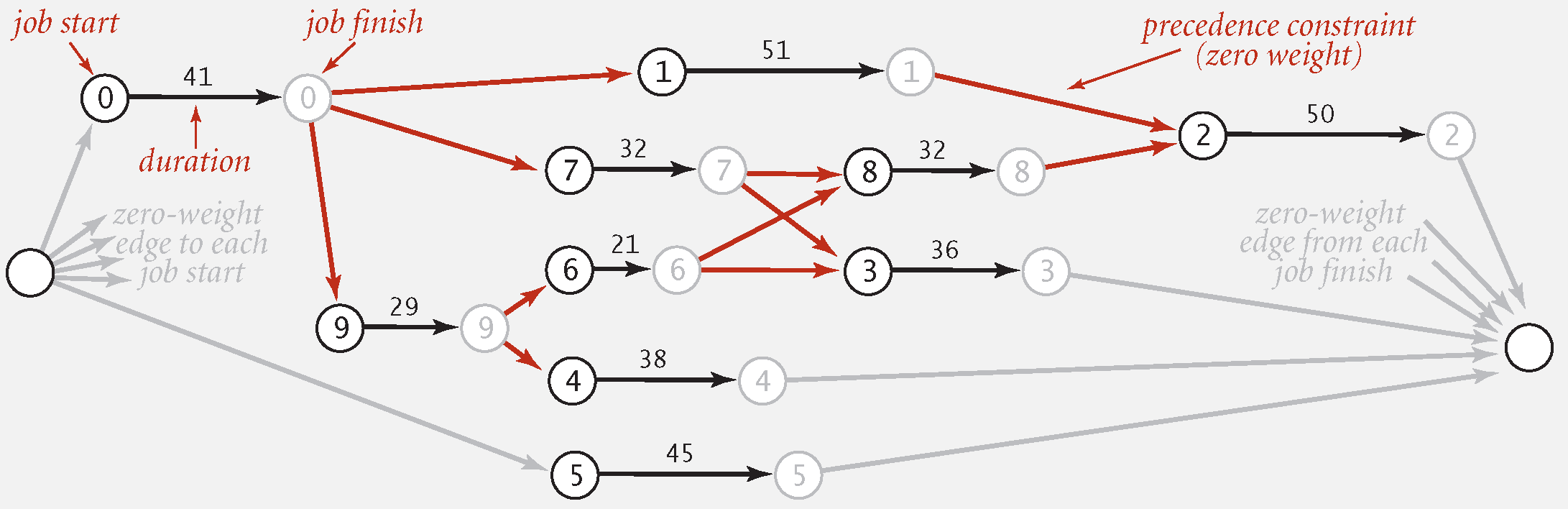
17.5 Negative Weights
Negative Cycle: A negative cycle is a directed cycle whose sum of edge weights is negative.
Bellman-Ford Algorithm
Initialize distTo[s] = 0 and distTo[v] = ∞ for all other vertices.
Repeat V times, relax each edge.
Practical Improvement: If distTo[v] does not change during pass
Algorithm | Restriction | Typical Case | Worst Case | Extra Space |
---|---|---|---|---|
Topological Sort | No Directed Cycles | |||
Dijkstra (Binary Heap) | No Negative Weights | |||
Bellman-Ford | No Negative Cycles | |||
Bellman-Ford (queue-based) |
Find A Negative Cycle
If there is a negative cycle, Bellman-Ford gets stuck in loop, updating distTo[] and edgeTo[] entries of vertices in the cycle.
If any vertex v is updated in phase V, there exists a negative cycle (and can trace back edgeTo[v] entries to find it).
Application - Arbitrage Detection
Currency exchange graph.
Vertex = currency.
Edge = transaction, with weight equal to exchange rate.
Find a directed cycle whose product of edge weights is > 1.
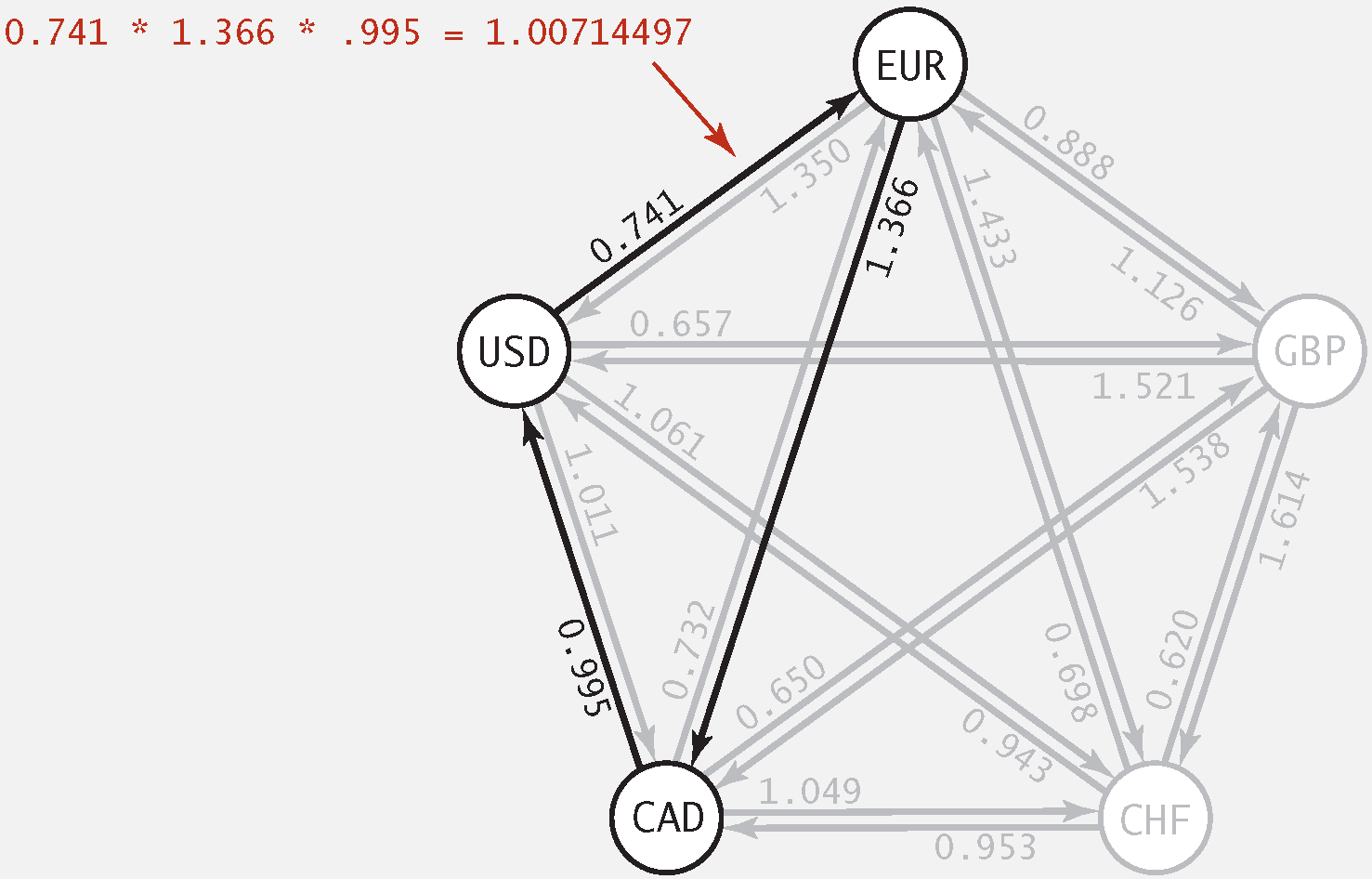
Arbitrage Detection
Let weight of edge
be (exchange rate from currency to ). Multiplication turns to addition;
turns to Find a directed cycle whose sum of edge weights is
(negative cycle).
18 Maximum Flow and Minimum Cut
18.1 Introduction
Definitions
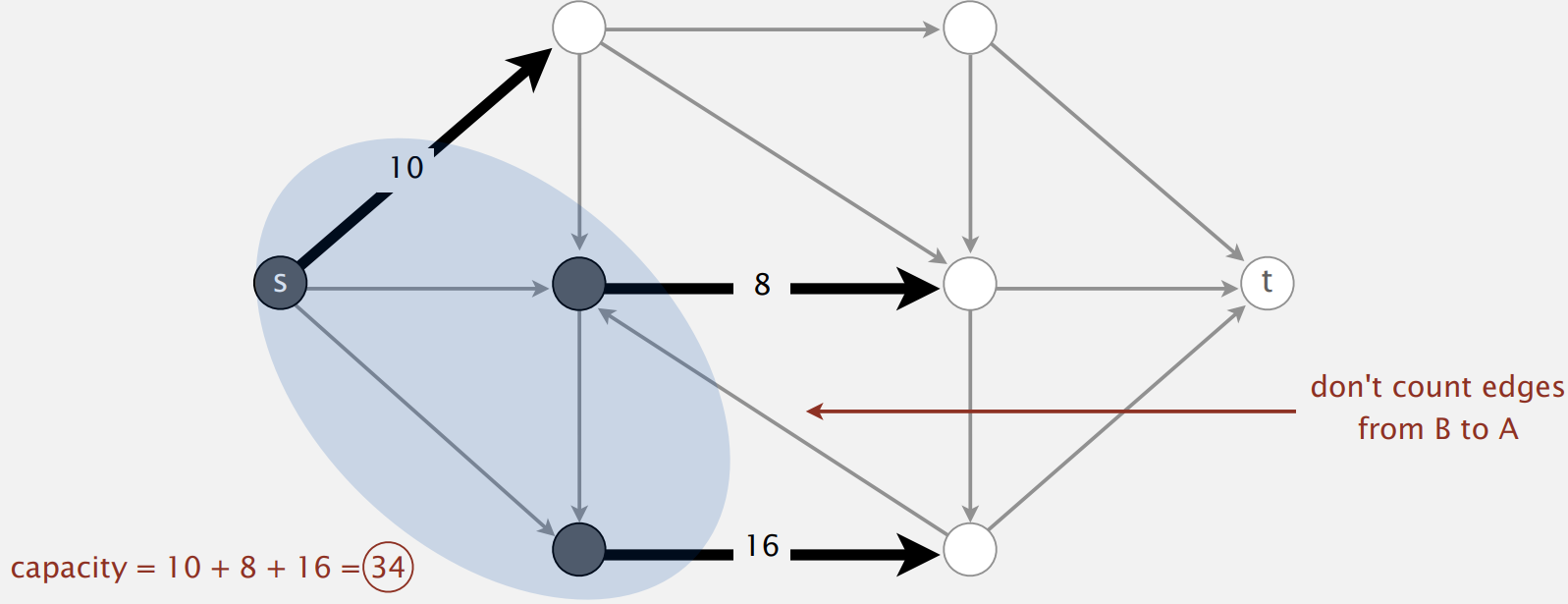
Minimum cut problem: Find a cut of minimum capacity.
Definitions
Capacity constraint: 0 ≤ edge's flow ≤ edge's capacity.
Local equilibrium: inflow = outflow at every vertex (except
and ).
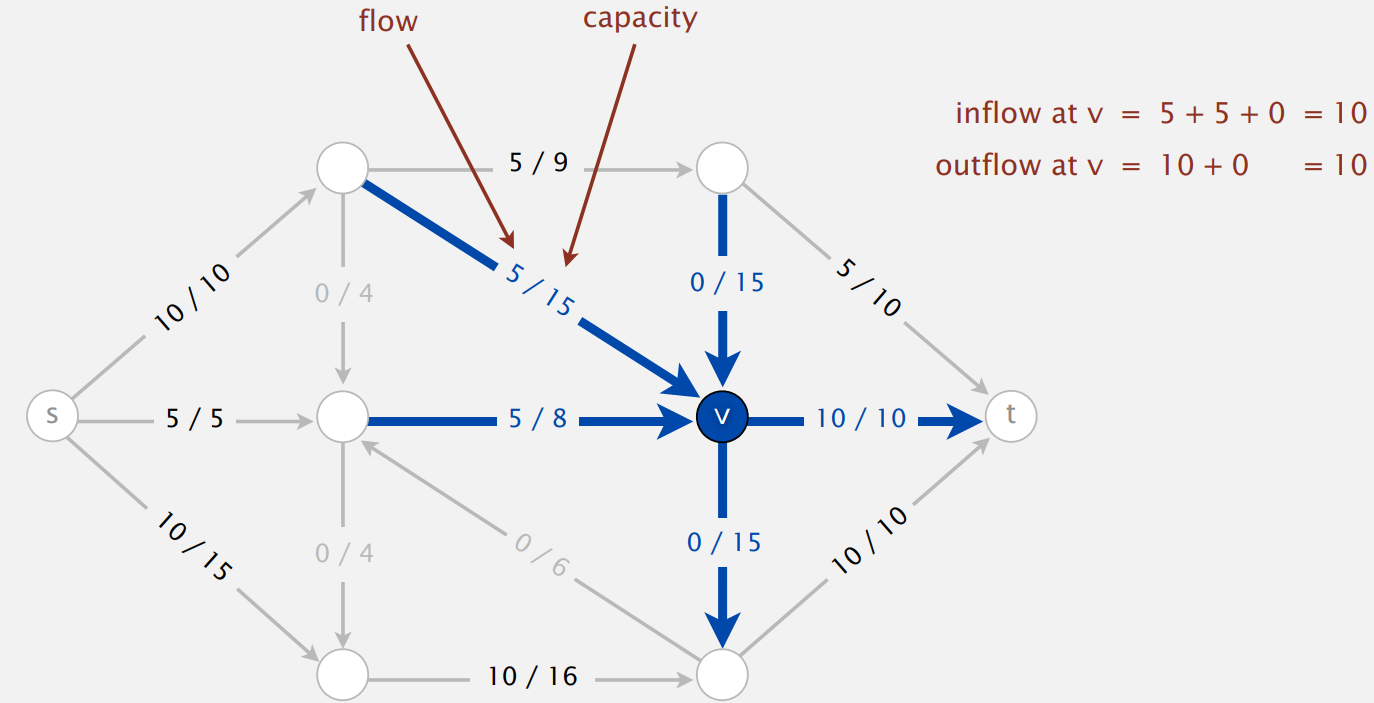
Value of a flow: The value of a flow is the inflow at
Maximum st-flow (maxflow) problem: Find a flow of maximum value.
18.2 Ford-Fulkerson Algorithm
Ford-Fulkerson Algorithm
Start with 0 flow.
Find an undirected path from s to t such that:
1. Can increase flow on forward edges (not full).
2. Can decrease flow on backward edges (not empty).
Terminates when all paths from s to t are blocked by either a full forward edge or an empty backward edge.
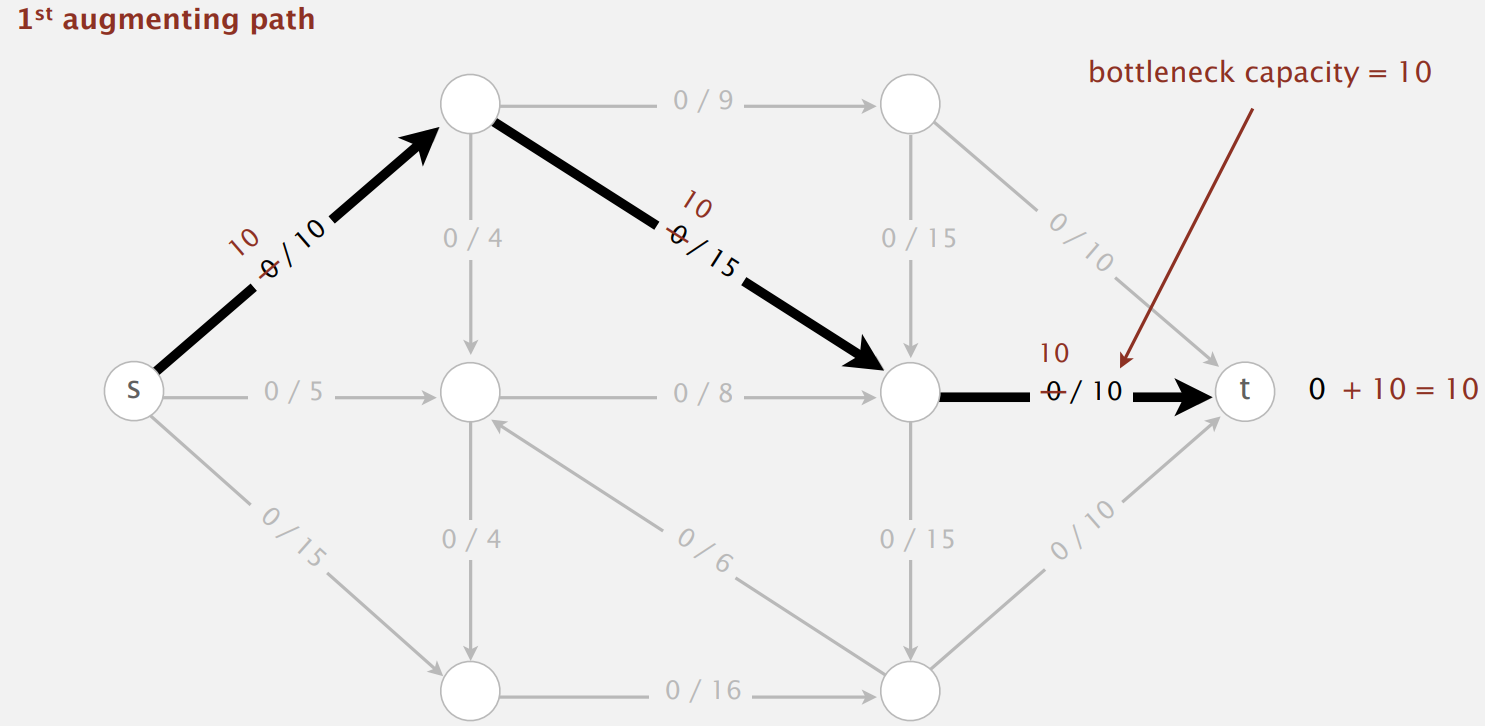
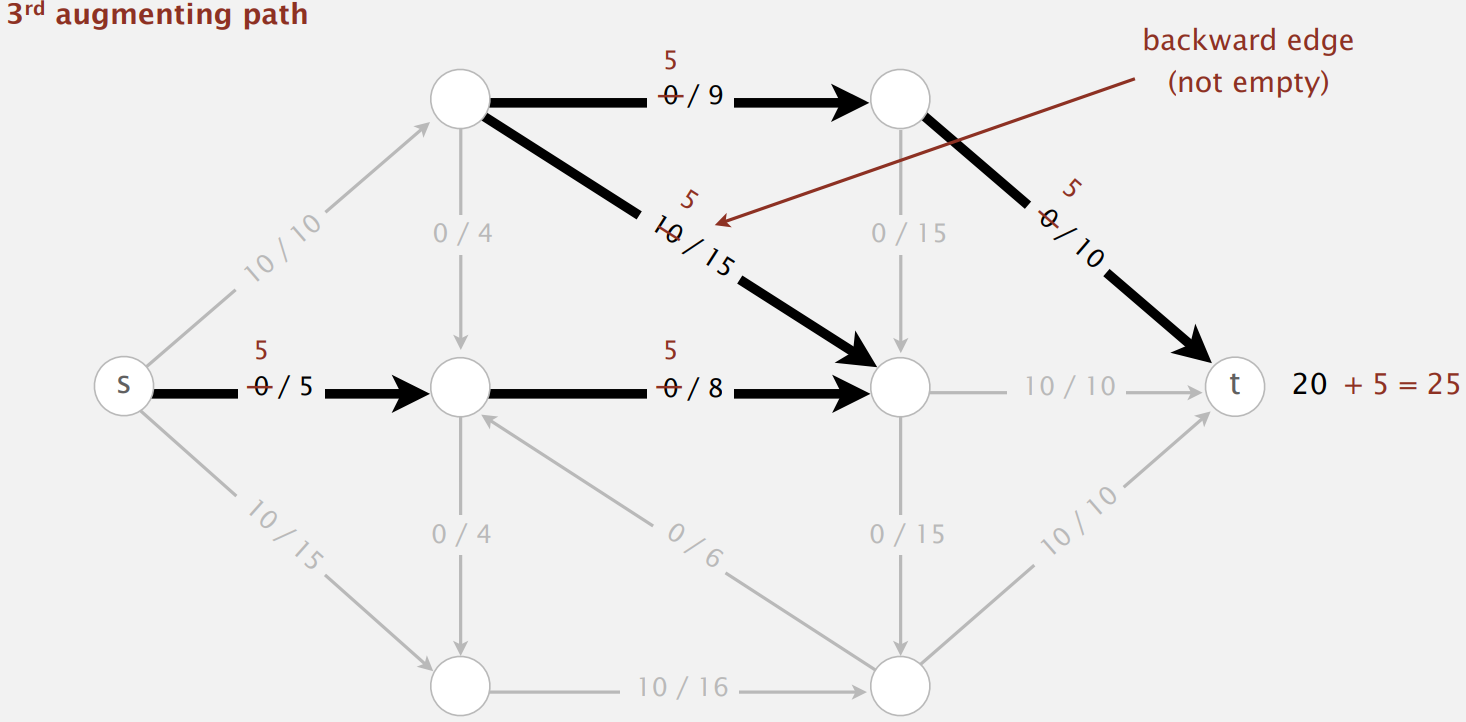
18.3 Maxflow-Mincut Theorem
Definition
Net Flow: The net flow across a cut (
Flow-value lemma: Let
Proof: By induction on the size of
Base case:
Induction step: remains true by local equilibrium when moving any vertex from
to .
Weak duality: Let
Proof
Value of flow
Augmenting path theorem: A flow f is a maxflow iff no augmenting paths.
Maxflow-mincut theorem: Value of the maxflow = capacity of mincut.
Proof: The following three conditions are equivalent for any flow
There exists a cut whose capacity equals the value of the flow
. is a maxflow. There is no augmenting path with respect to
.
1 -> 2
Suppose that
is a cut with capacity equal to the value of . Then, the value of any flow
≤ capacity of = value of . Thus,
is a maxflow.
2 -> 3: We prove contrapositive: ~3 -> ~2
Suppose that there is an augmenting path with respect to
. Can improve flow
by sending flow along this path. Thus, f is not a maxflow.
3 -> 1: Suppose that there is no augmenting path with respect to
Let
be a cut where is the set of vertices connected to by an undirected path with no full forward or empty backward edges. By definition,
is in ; since no augmenting path, is in . Capacity of cut = net flow across cut (forward edges full; backward edges empty) = value of flow
(flow-value lemma).
To compute mincut
By augmenting path theorem, no augmenting paths with respect to
. Compute
= set of vertices connected to by an undirected path with \ no full forward or empty backward edges.
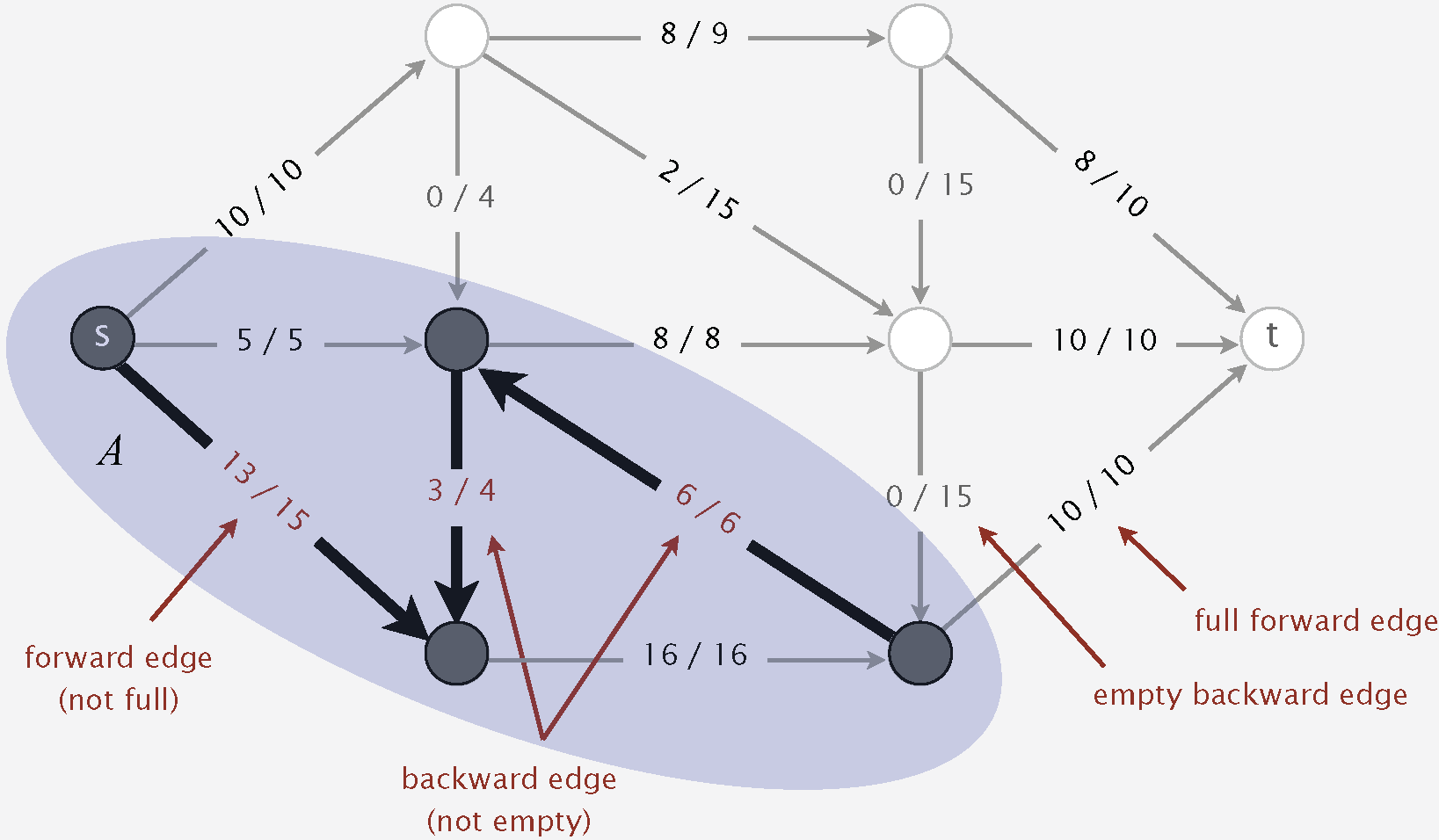
18.4 Running Time Analysis
Properties
The flow is integer-valued throughout Ford-Fulkerson.
Proof
Bottleneck capacity is an integer.
Flow on an edge increases/decreases by bottleneck capacity.
Number of augmentations ≤ the value of the maxflow.
Proof: Each augmentation increases the value by at least 1.
Integrality theorem: There exists an integer-valued maxflow.
Proof: Ford-Fulkerson terminates and maxflow that it finds is integer-valued.
Running time: FF performance depends on choice of augmenting paths.
Digraph with
Augmenting Path | Number of Paths | Implementation |
---|---|---|
Shortest Path | Queue (BFS) | |
Fattest path | Priority Queue | |
Random Path | Randomized Queue | |
DFS Path | Stack (DFS) |
18.5 Implementation
18.5.1 Flow Edge
Implementation
Use residual capcity:
Forward edge: residual capacity
. Backward edge: residual capacity
.
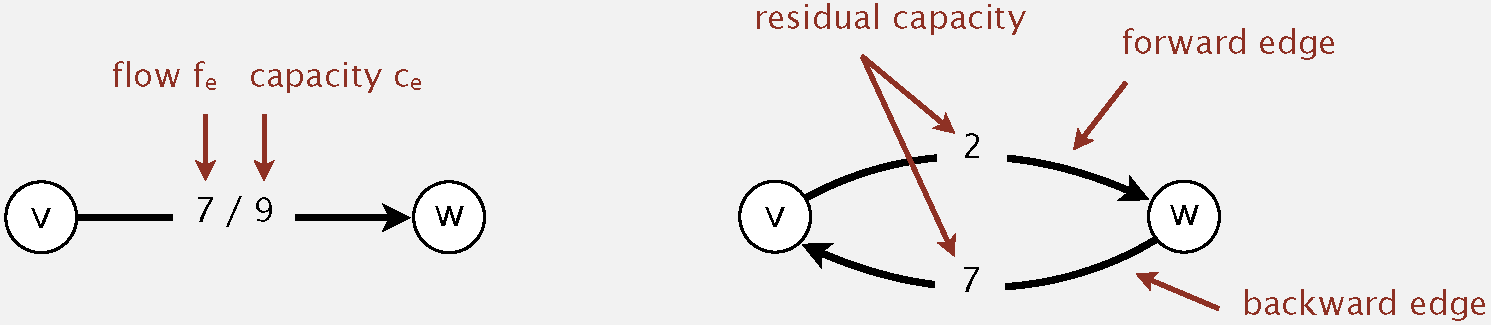
18.5.2 Flow Network
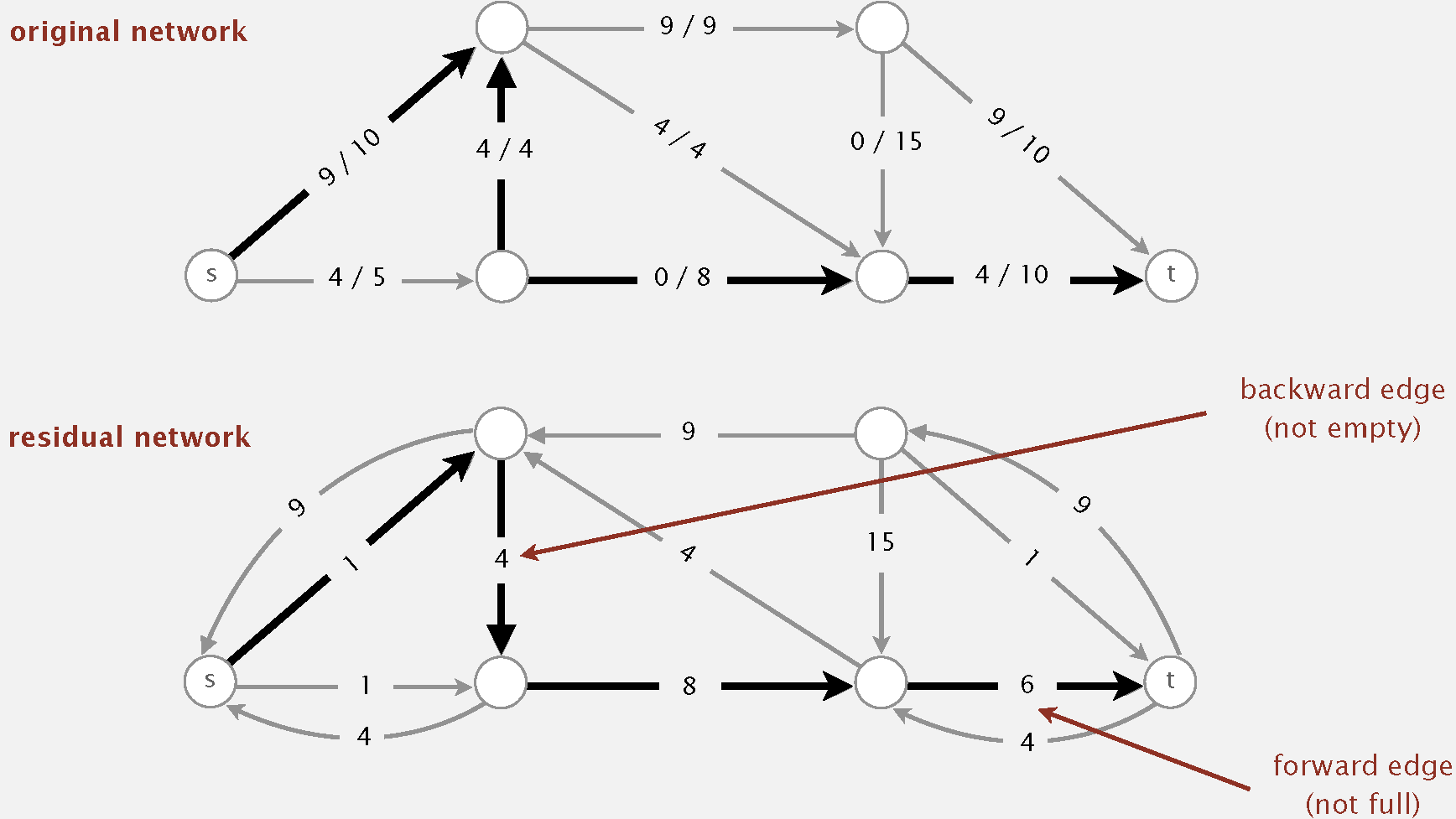
18.5.3 Ford-Fulkerson Algorithm
18.6 Maxflow Applications
Applications
Data mining.
Open-pit mining.
Bipartite matching.
Network reliability.
Baseball elimination.
Image segmentation.
Network connectivity.
Distributed computing.
Security of statistical data.
Egalitarian stable matching.
Multi-camera scene reconstruction.
Sensor placement for homeland security.
Many, many, more.
18.6.1 Bipartite Matching
N students apply for N jobs. Given a bipartite graph, find a perfect matching.
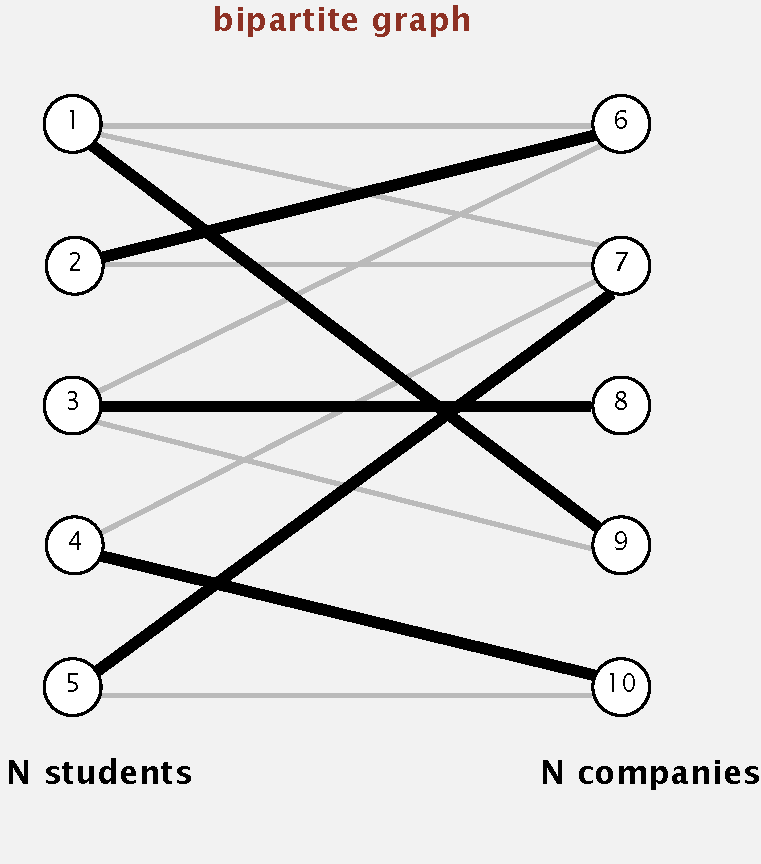
Bipartite Matching
Create
, , one vertex for each student, and one vertex for each job. Add edge from
to each student (capacity 1). Add edge from each job to
(capacity 1). Add edge from student to each job offered (infinite capacity).
1-1 correspondence between perfect matchings in bipartite graph and integer-valued maxflows of value
.
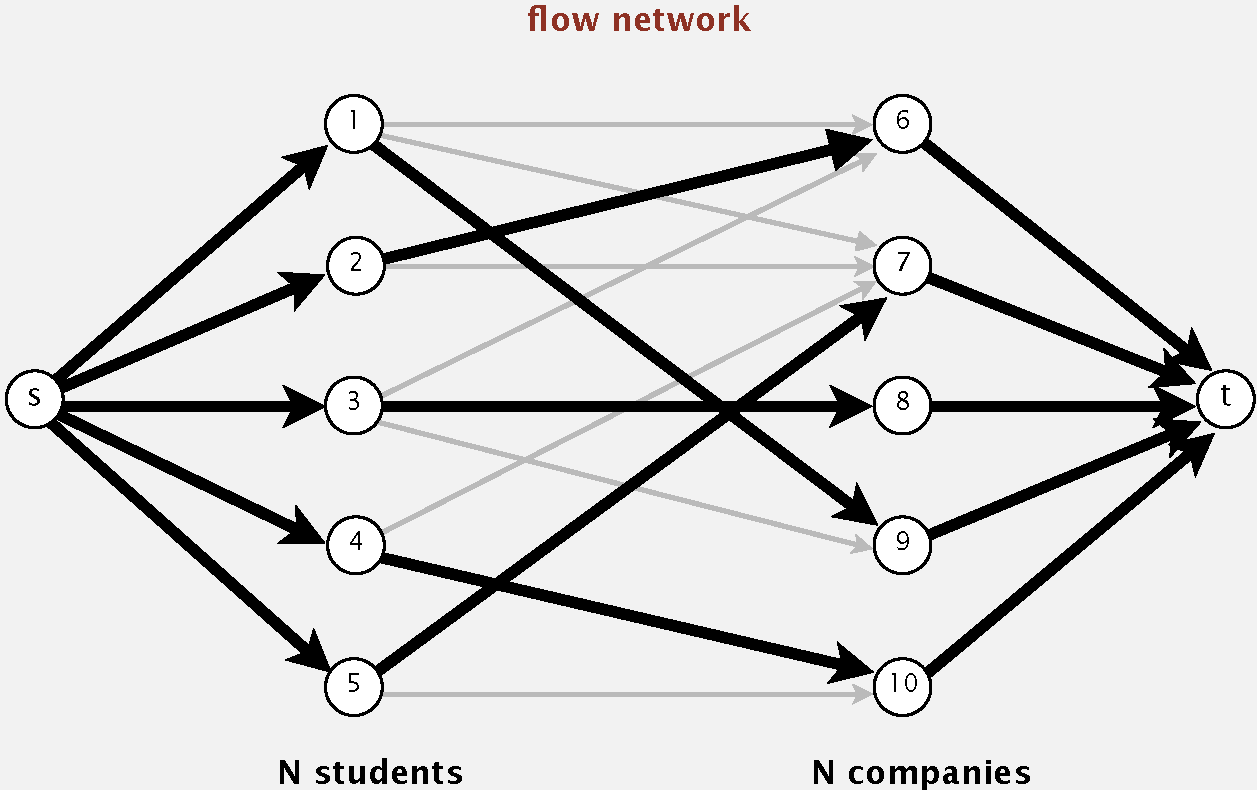
When no perfect matching, mincut explains why
Consider mincut (
Let
= students on side of cut. Let
= companies on side of cut. Fact:
; students in can be matched only to companies in .
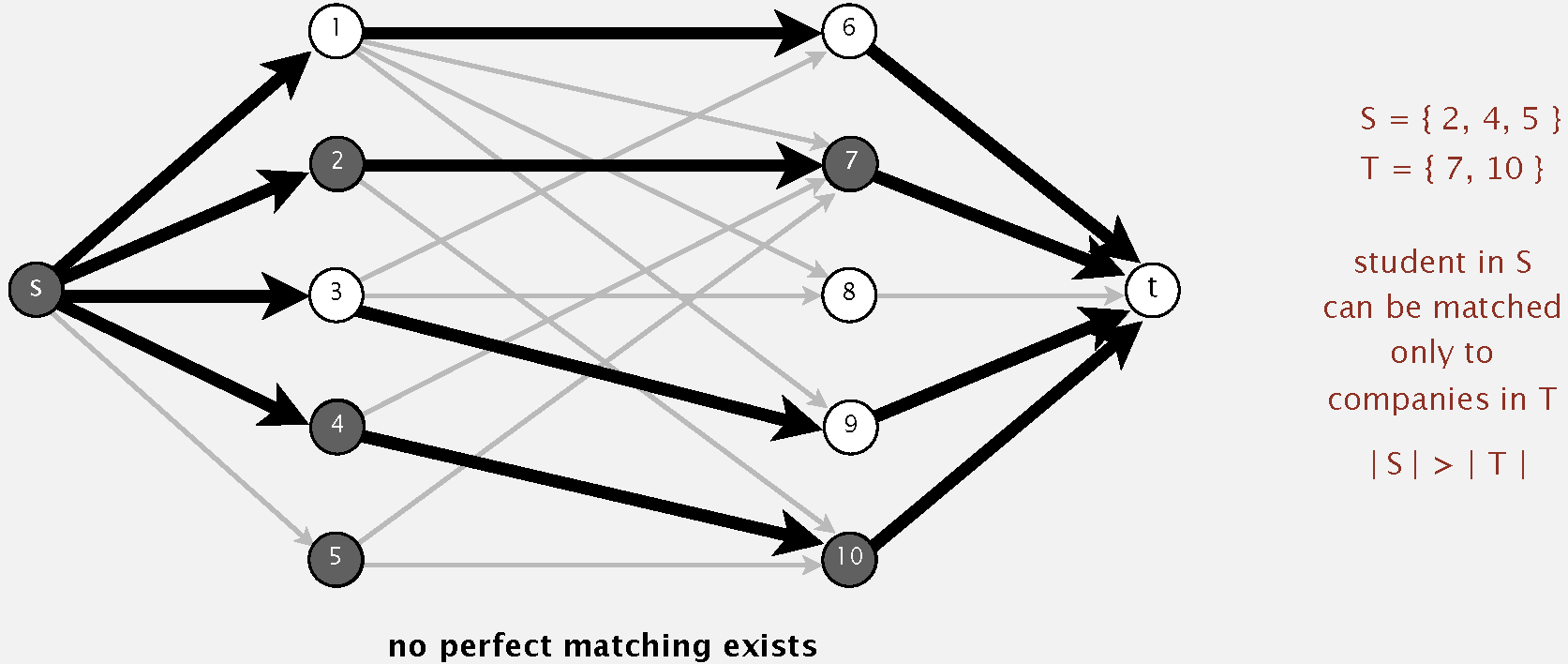
18.6.2 Baseball Elimination
Problem: Which teams have a chance of finishing the season with the most wins?
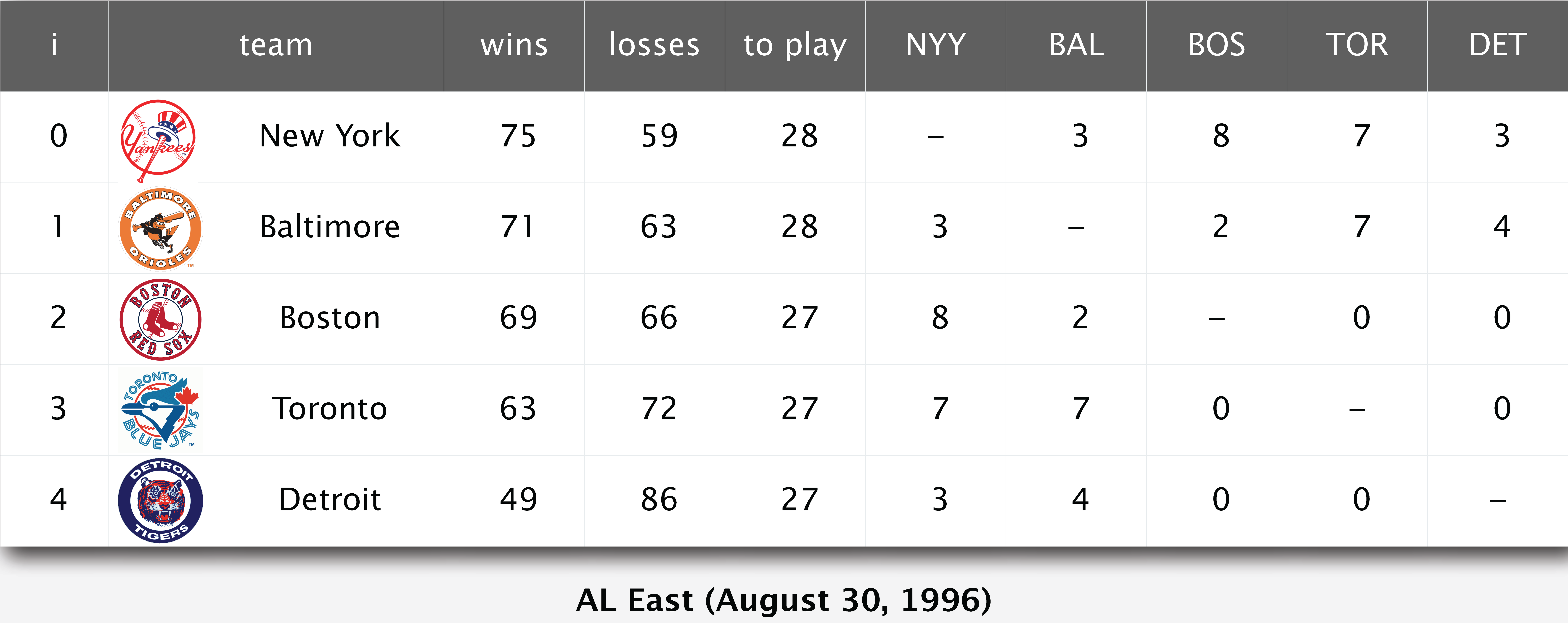
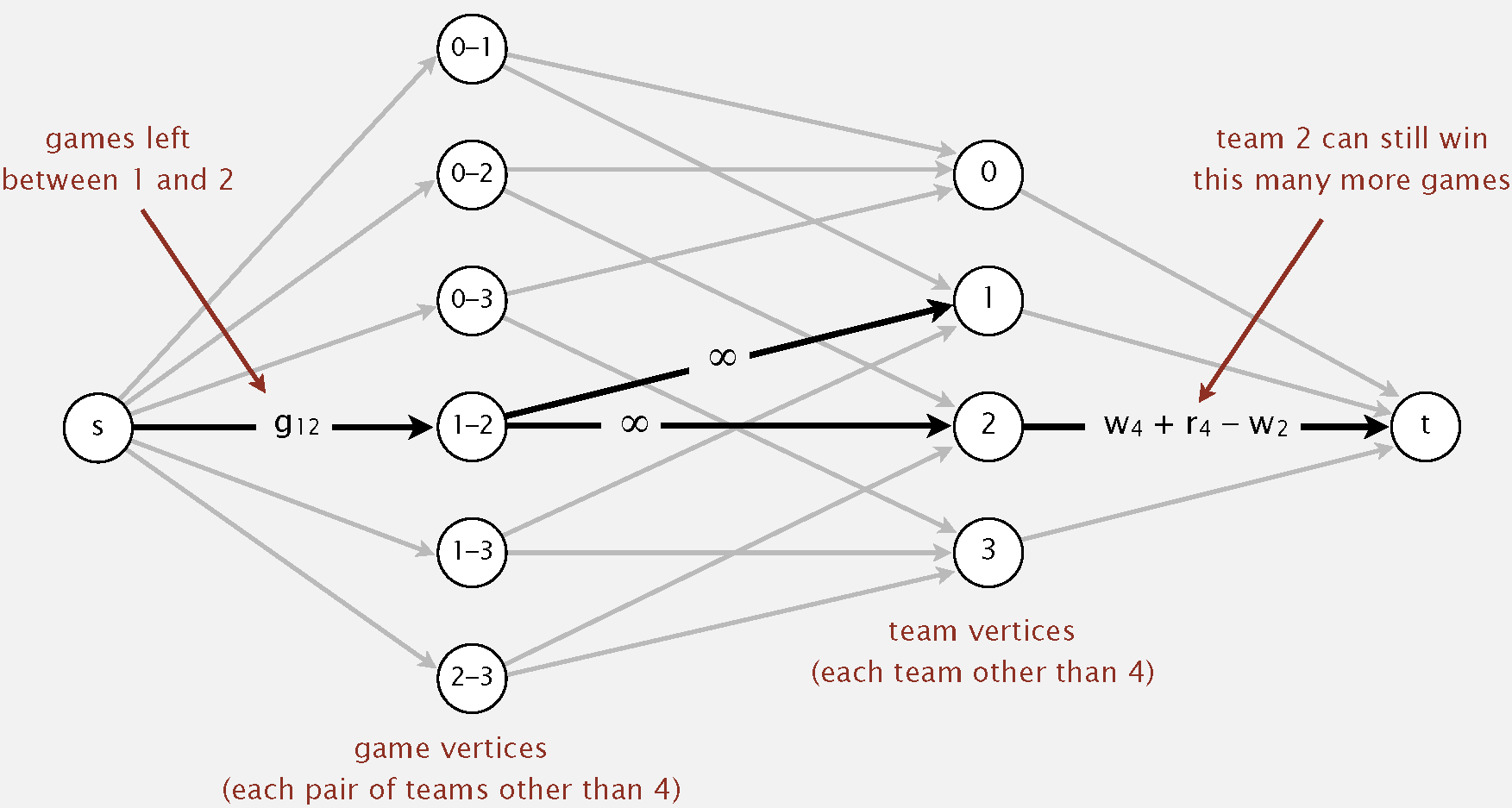
Team 4 not eliminated iff all edges pointing from s are full in maxflow.
19 Radix Sort
19.1 Strings in Java
String: Sequence of characters.
The char data type
C char data type: Typically an 8-bit integer.
Supports 7-bit ASCII.
Can represent only 256 characters.
Java char data type: A 16-bit unsigned integer.
Supports original 16-bit Unicode.
Supports 21-bit Unicode 3.0 (awkwardly).
Examples
String | StringBuilder | ||||
---|---|---|---|---|---|
Data Type in Java | Sequence of characters (immutable) | Sequence of characters (mutable) | |||
Underlying Implementation | Immutable | Resizing | |||
Guarantee | Extra Space | Guarantee | Extra Space | ||
Operation |
| ||||
| |||||
| |||||
|
19.2 Key-Indexed Counting
Assumption: Keys are integers between
Goal: Sort an array of
Key-Indexed Counting
Count frequencies of each letter using key as index.
Compute frequency cumulates which specify destinations.
Access cumulates using key as index to move items.
Copy back into original array.
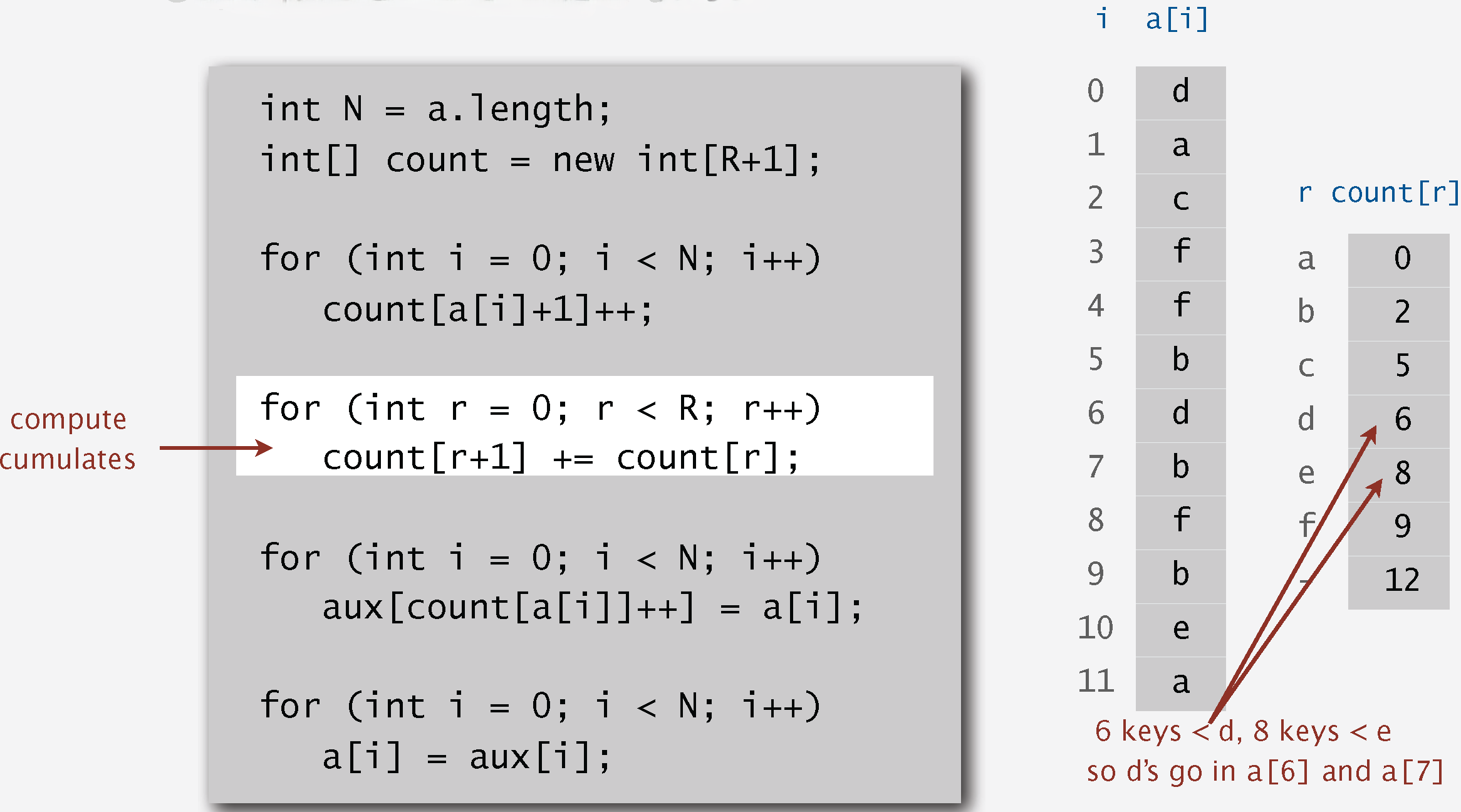
Properties
Key-indexed counting uses
array accesses to sort items whose keys are integers between and . Key-indexed counting uses extra space proportional to
. Key-indexed counting is stable.
19.3 LSD Radix Sort
LSD Radix Sort
Consider characters from right to left.
Stably sort using dth character as the key (using key-indexed counting).
Correctness Proof: LSD sorts fixed-length strings in ascending order.
Proof
After pass
If two strings differ on sort key, key-indexed sort puts them in proper relative order.
If two strings agree on sort key, stability keeps them in proper relative order.
Property: LSD sort is stable.
Google (or presidential) Interview Question: Sort one million 32-bit integers using LSD radix sort.
19.4 MSD Radix Sort
MSD Radix Sort
Partition array into
pieces according to first character (use key-indexed counting ). Recursively sort all strings that start with each character (key-indexed counts delineate subarrays to sort).
Variable-length strings: Treat strings as if they had an extra char at end (smaller than any char)
Potential for Disastrous Performance
Much too slow for small subarrays.
Each function call needs its own
count[]
array.ASCII (256 counts): 100x slower than copy pass for
. Unicode (65,536 counts): 32,000x slower for
.
Huge number of small subarrays because of recursion.
Improvements
Cutoff to insertion sort for small subarrays.
Insertion sort, but start at
character. Implement
less()
so that it compares starting atcharacter.
Performance
Number of characters examined.
MSD examines just enough characters to sort the keys.
Number of characters examined depends on keys.
Can be sublinear in input size!
MSD String Sort vs. Quicksort for Strings
Disadvantages of MSD string sort:
Extra space for aux[] arrays.
Extra space for count[] arrays.
Inner loop has a lot of instructions.
Accesses memory "randomly" (cache inefficient).
Disadvantage of quicksort
Linearithmic number of string compares (not linear).
Has to rescan many characters in keys with long prefix matches .
19.5 3-Way Radix Quicksort
Do 3-way partitioning on the
Properties
Less overhead than R-way partitioning in MSD string sort.
Does not re-examine characters equal to the partitioning char.
(but does re-examine characters not equal to the partitioning char)
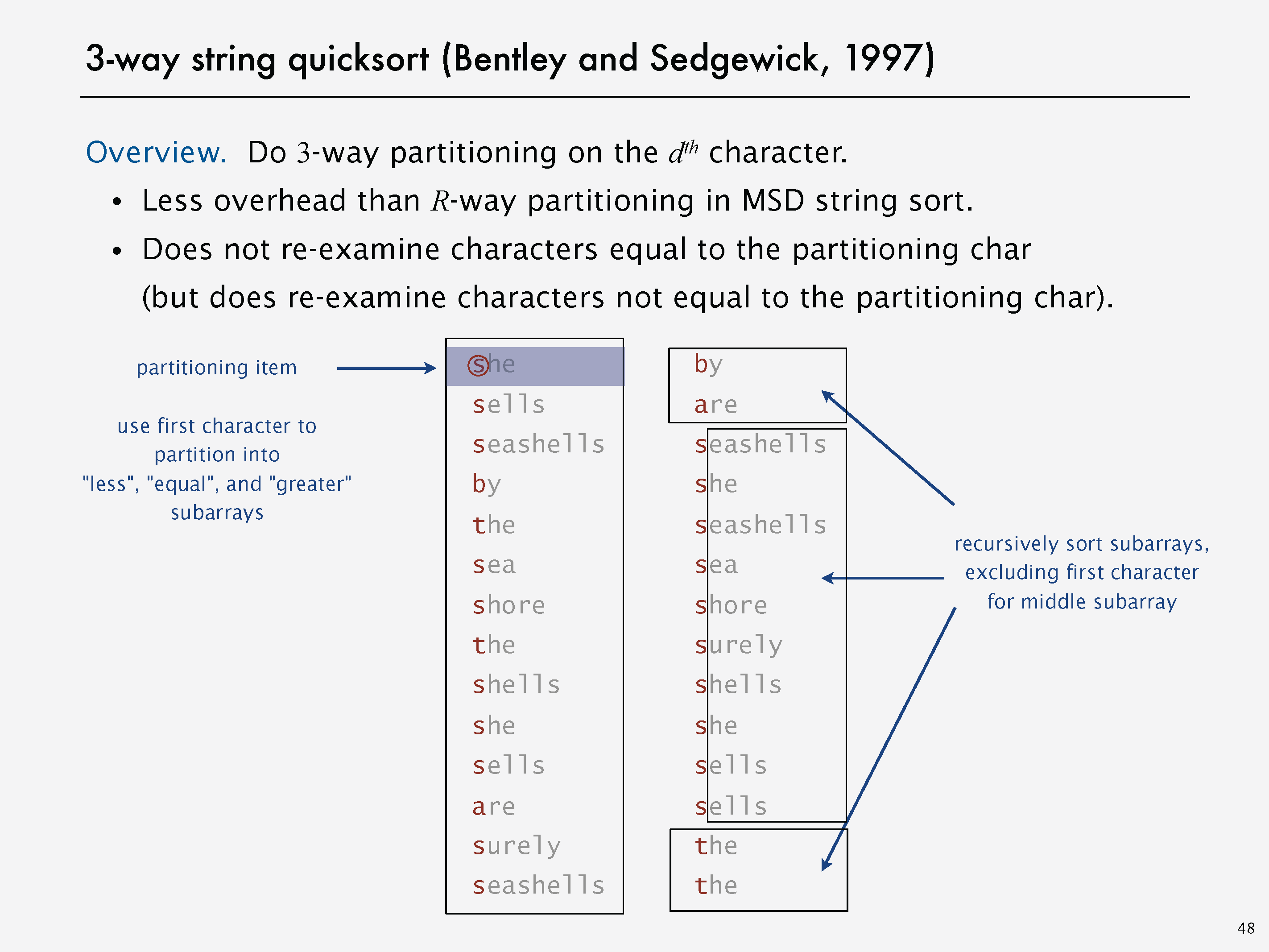
3-Way String Quicksort vs. Standard Quicksort
Standard quicksort
Uses
string compares on average. Costly for keys with long common prefixes (and this is a common case!)
3-way string (radix) quicksort
Uses
character compares on average for random strings. Avoids re-comparing long common prefixes.
3-way String Quicksort vs. MSD String Sort
MSD string sort
Is cache-inefficient.
Too much memory storing count[].
Too much overhead reinitializing count[] and aux[].
3-way string quicksort
Has a short inner loop.
Is cache-friendly.
Is in-place.
Summary of the Performance of Sorting Algorithms
Algorithm | Guarantee | Random | Extra Space | Stable? | Operations on keys |
---|---|---|---|---|---|
Yes |
| ||||
Yes |
| ||||
No |
| ||||
No |
| ||||
Yes |
| ||||
Yes |
| ||||
No |
|
*: probabilistic
☆: fixed-length W keys
★: average-length W keys
19.6 Suffix Arrays
Keyword in context search: Given a text of N characters, preprocess it to enable fast substring search (find all occurrences of query string context).
Applications: Linguistics, databases, web search, word processing, ...
Keyword-in-context search: suffix-sorting solution.
Preprocess: suffix sort the text.
Query: binary search for query; scan until mismatch.