RISC-Ⅴ Architecture
6 Introduction to Assembly Language
6.1 Assembly Language
Assembly (also known as Assembly language, ASM): A low-level programming language where the program instructions match a particular architecture's operations.
Architecture: (also ISA: instruction set architecture) The parts of a processor design that one needs to understand for writing assembly/machine code.
Properties:
Splits a program into many small instructions that each do one single part of the process.
Each architecture will have a different set of operations that it supports (although there are similarities).
Assembly is not portable to other architectures.
Complex/Reduced Instruction Set Computing
Early trend - add more and more instructions to do elaborate operations
Complex Instruction Set Computing (CISC)
Difficult to learn and comprehend language
Less work for the compiler
Complicated hardware runs more slowly
Opposite philosophy later began to dominate
Reduced Instruction Set Computing (RISC)
Simple (and smaller) instruction set makes it easier to build fast hardware.
Let software do the complicated operations by composing simpler ones.
Code:
op dst, src1, src2
op
: operation name ("operator")dst
: register getting result ("destination")src1
: first register for operation ("source 1")src2
: second register for operation ("source 2")
6.2 Registers
Assembly uses registers to store values. Registers are:
Small memories of a fixed size.
Can be read or written.
Limited in number.
Very fast and low power to access.
Registers | Memory | |
---|---|---|
Speed | Fast | Slow |
Size | Small e.g., 32 registers * 32 bit = 128 bytes | Big 4-32 GB |
Connection | More variables than registers? Keep most frequently used in registers and move the rest to memory |
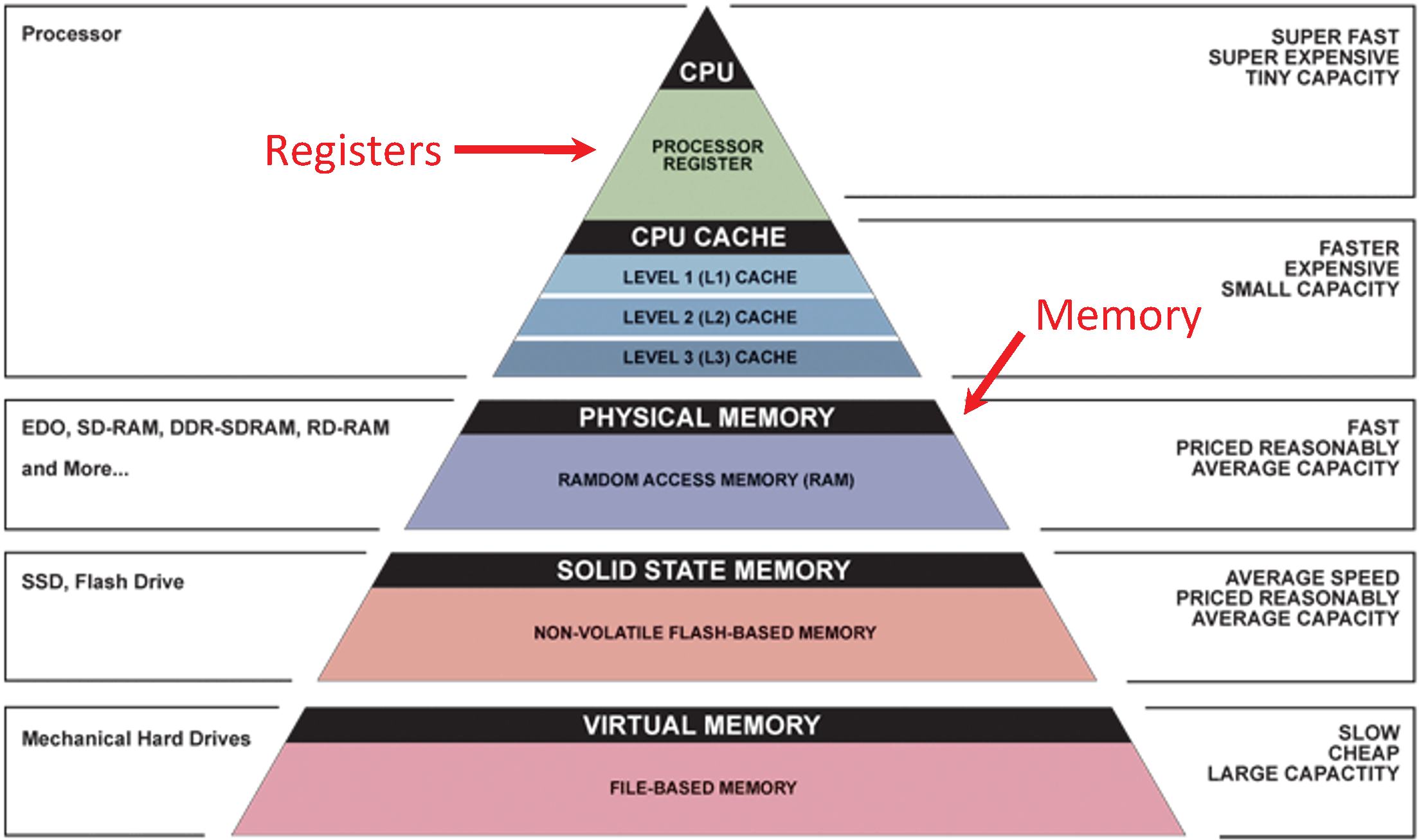
6.3 RISC-Ⅴ Instructions
In high-level languages, variable types determine operation.
In assembly, operation determines type, i.e., how register contents are treated.
Operations
Transfer data between memory and register
Load data from memory into register
Store register data into memory
Perform arithmetic function on register or memory data
Transfer control
Unconditional jumps to/from procedures
Conditional branches
Indirect branches
6.3.1 Basic Arithmetic Instructions
Types:
Integer Addition
C: a = b + c;
RISC-Ⅴ: add s1, s2, s3
Integer Subtraction
C: a = b - c;
RISC-Ⅴ: sub s1, s2, s3
6.3.2 Immediate Instructions
Immediates: Numerical constants.
Syntax: opi dst, src, imm
Operation names end with "i", replace
source register with an immeidate. Immediates can up to 12-bits in size.
Interpreted as sign-extended two's complement.
RISC-Ⅴ hardwires the register zero (x0) to value 0.
Example: RISC-Ⅴ: add x3 x4 0
C: f = g
6.3.3 Data Transfer Instructions
Specialized data transfer instructions move data between registers and memory.
Store: register TO memory
Load: register FROM memory
Syntax: memop reg, off (bAbbr)
memop
: operation name ("operator")reg
: register for operation source or destination.bAbbr
: register with pointer to memory ("base address")off
: Address offset (immediate) in bytes ("offset")
Types:
Load Word: Takes data at address
bAbbr+off
FROM memory and places it intoreg
.Store Word: Takes data in
reg
and stores it TO memory atbAbbr+off
.
Example: address of int array [] -> s3, value of b -> s2
C: array[10] = array[3] + b;
RISC-Ⅴ
lw t0, l2 (s3) # t0 = A[3]
add t0, s2, t0 # t0 = A[3] + b
sw t0, 40 (s3) # A[10] = A[3] + b
6.3.4 Control Flow Instructions
Labels in RISC-Ⅴ: Defined by a text and followed by a colon (e.g., main:) and refers to the instructions that follows; generate control flow by jumping to labels.
Types:
Branch If Equal (beq)
Syntax: beq reg1, reg2, label
If value in reg1 == value in reg2, go to label.
Otherwise go to next instruction.
Branch If Not Equal (bne)
Syntax: bne reg1, reg2, label
If value in reg1 ≠ value in reg2, go to label.
Jump (j)
Syntax: j label
Unconditional jump to label.
Branch Less Than (blt)
Syntax: blt reg1, reg2, label
If value in reg1 < value in reg2, go to label.
Branch Less Than or Equal (ble)
Syntax: ble reg1, reg2, label
If value in reg1 ≤ value in reg2, go to label.
Loops in RISC-Ⅴ:
There are three types of loops in C: while, do...while, and for.
These can be created with branch instructions as well.
The key to decision making is the branch statement.
Program Counter:
Program Counter (PC): A special register that contains the current address of the code that is being executed.
Branches and Jumps change the flow of execution by modifying the PC.
Instructions are stored as data in memory (code section) and have addresses! Labels get converted to instruction addresses.
The PC tracks where in memory the current instruction is.
6.3.5 Shifting Instructions
Shifting Operations
Instruction Name | RISC-Ⅴ |
---|---|
Shift Left Logical | sll s1, s2, s3 |
Shift Left Logical Imm | sll1 s1, s2, imm |
Shift Right Logical | srl s1, s2, s3 |
Shift Right Logical Imm | srli s1, s2, imm |
Shift Right Arithmetic | sra s1, s2, s3 |
Shift Right Arithmetic Imm | srai s1, s2, imm |
6.4 C, Assembly & Machine Code
Compile with the following code (debugging-friendly):
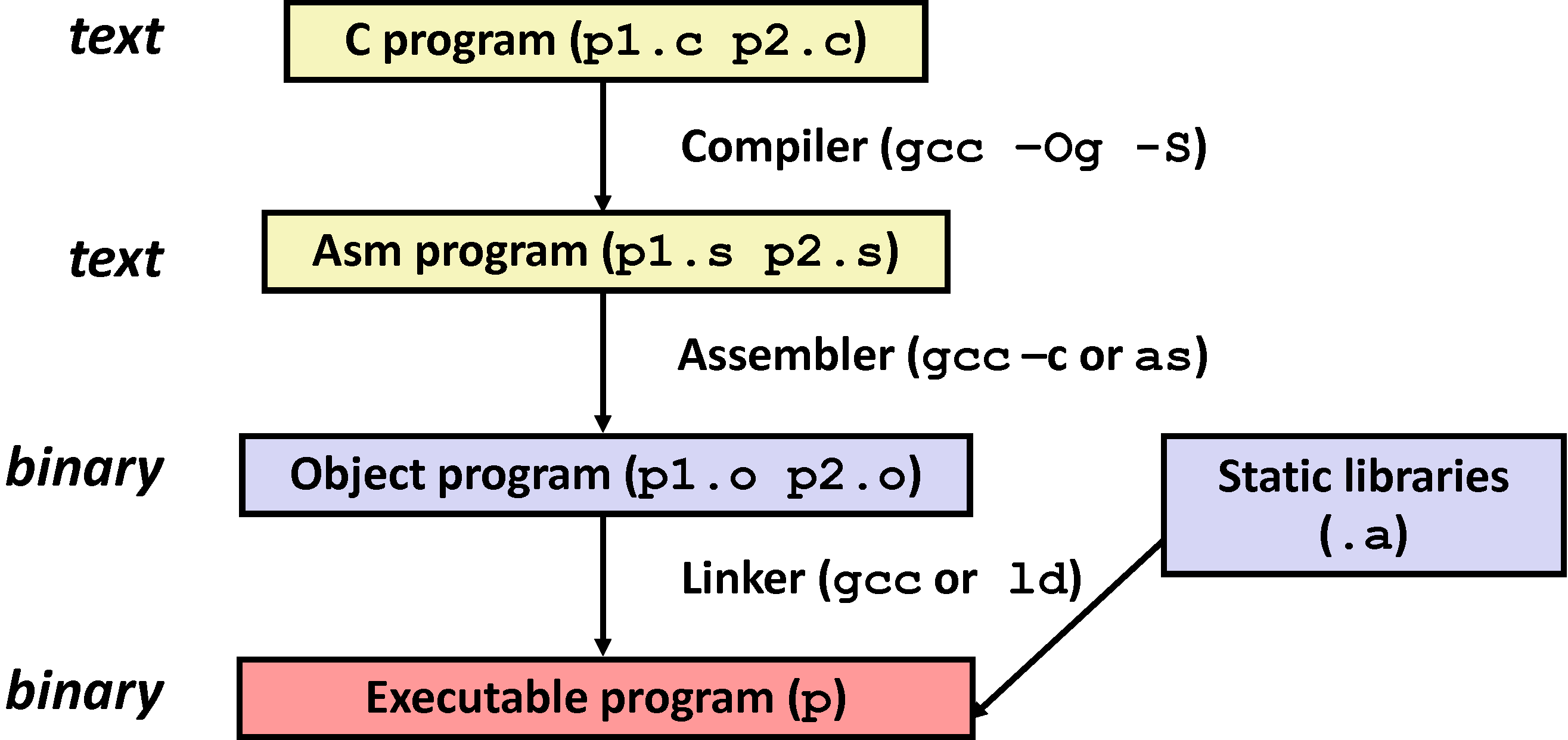
Use the following command to generate the assembly code:
Use the following command to disassembly the machine code: