Computer Architecture
Last Modified: 4/10/2025
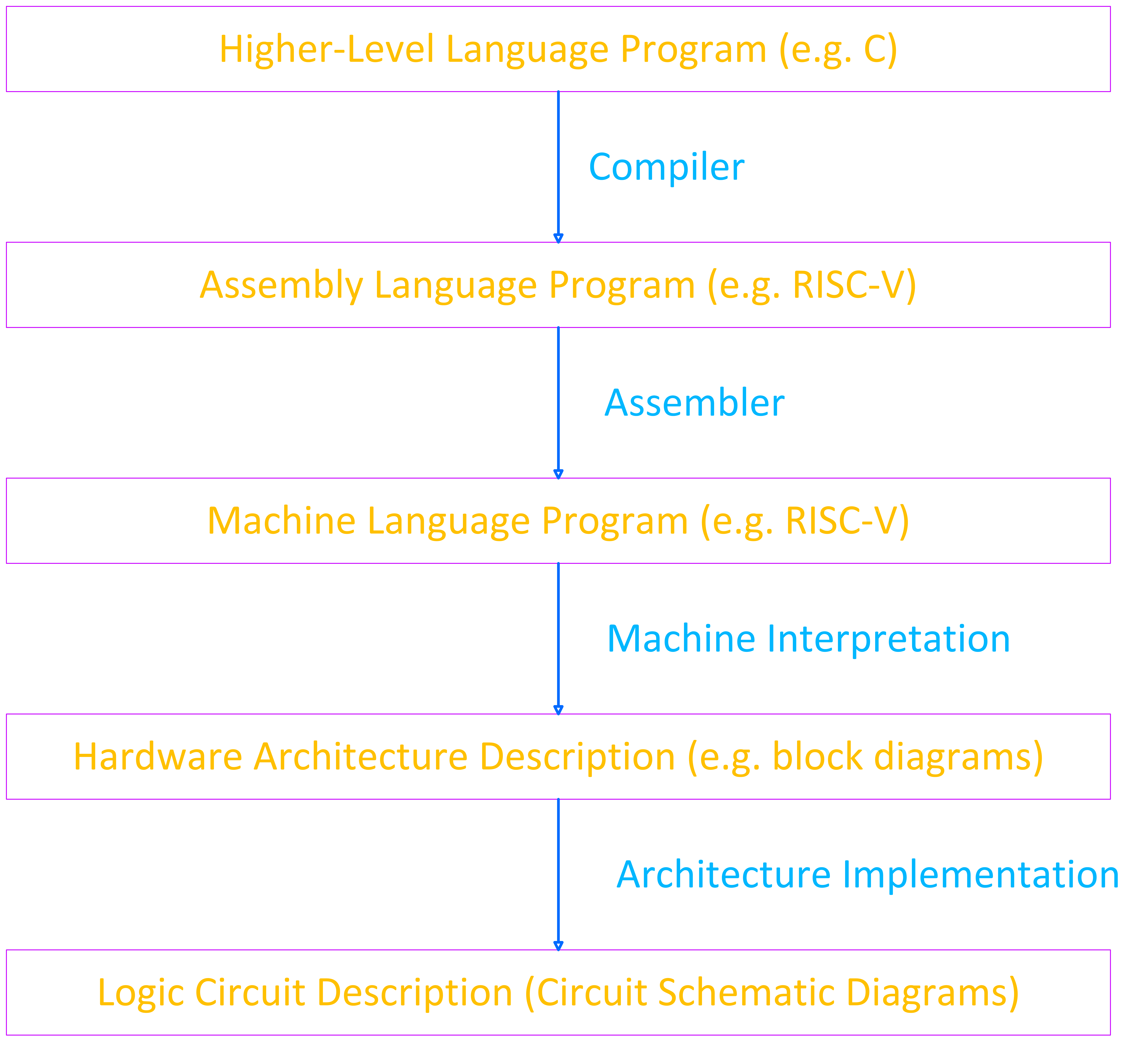
In this part, we will explore computer architecture, both x86-64 and RISC-V architecture.
1 Bit, Bytes & Number Representation
1.1 Number Base
In computer science, there are three commonly-used number bases: binary, decimal & hexadecimal.
- Binary (base 2)
- Symbols: 0, 1
- Notation:
- Converting numbers to base 2 lets us represent numbers as bits!
- Decimal (base 10)
- Symbols: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
- Notation:
- Understandable by humans, used in our daily life.
- Hexadecimal (base 16)
- Symbols: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, A, B, C, D, E, F
- Notation:
- A convenient shorthand for writing long sequences of bits.
Conversion between bases
-
Convert from other bases to base-10: write out power of bases.
For example,
-
Convert from base-10 to other bases: Use the “leftover algorthm”
For example, convert to base-4. For base-4, the powers of base include 256, 64, 16, 4, 1.
- How many multiples of fit in ? left over.
- How many multiples of fit in ? Still left over.
- How many multiples of fit in ? left over.
- How many multiples of fit in ? , which means we are done!
Therefore, .
When converting between different bases, you can refer to the following table below.
Decimal | Binary | Hexadecimal |
---|---|---|
1.2 Integer Representation
1.2.1 Unsigned Integers
Properties
- Idea: Simply convert decimal to binary, cCan represent different numbers!
- Smallest number: represents .
- Largest number: represents .
Conclusion
Unsigned Integer | |
---|---|
Can represent negative numbers | ✘ |
Doing math is easy | ✔ |
Every bit sequence represents a unique number | ✔ |
1.2.2 Signed Integers
Properties
- Idea: Use the left-most bit to indicate if the number is positive (0) or negative (1). This is called sign-magnitude representation.
- Smallest number: represents .
- Largest number: represents .
Sign-Magnitude | |
---|---|
Can represent negative numbers | ✔ |
Doing math is easy | ✘ |
Every bit sequence represents a unique number | ✘ |
1.2.3 One’s Complement
Properties
- Idea: If the number is negative, flip the bits. For example, is , so is . Left-most bit acts like a sign bit. If it’s 1, someone flipped the bits, so number must be negative.
- Smallest number: represents .
- Largest number: represents .
One’s Complement | |
---|---|
Can represent negative numbers | ✔ |
Doing math is easy | ✔ |
Every bit sequence represents a unique number | ✘ |
1.2.4 Two’s Complement
Properties
- Idea: If the number is negative, flip the bits, and add one (because we shift to avoid double-zero). Because of overflow, addition behaves like modular arithmetic (For example, and are the same in land: ).
- Smallest number: represents .
- Largest number: represents .
Conversion between two’s complement and signed integer
-
Two’s Complement -> Signed Integer
- If left-most digit is 0: Read it as unsigned
- If left-most digit is 1:
- Flip the bits, and add 1
- Convert to base-10, and stick a negative sign in front
Example: What is in decimal?
- Flip the bits:
- Add one:
- In base-10:
-
Signed Integer -> Two’s Complement
- If number is positive: Just convert it to base-2
- If number is negative:
- Pretend it’s unsigned, and convert to base-2
- Flip the bits, and add 1
Example: What is in two’s complement binary?
- In base-2:
- Flip the bits:
- Add one:
Two’s Complement | |
---|---|
Can represent negative numbers | ✔ |
Doing math is easy | ✔ |
Every bit sequence represents a unique number | ✔ |
1.2.5 Bias Notation
Properties
- Idea: Just like unsigned, but shifted on the number line.
- Smallest number: represents .
- Largest number: represents .

Bias Notation | |
---|---|
Can represent negative numbers | ✔ |
Doing math is easy | ✘ |
Every bit sequence represents a unique number | ✔ |
1.2.6 Sign Extension
For binary representation, leftmost bit is the most significant bit (MSB), and rightmost bit is the least significant bit (LSB).
- Idea: Want to represent the same number using more bits than before. It’s easy for positive numbers (add leading 0’s), but more complicated for negative numbers.
- Sign and magnitude: Add 0’s after the sign bit.
- One’s/Two’s complement: Copy MSB.
Example
- Sign and magnitude:
- One’s complement:
2 C Introduction
2.1 Variable C Types
2.2 Addresses & Pointers
A computer memory location has an address and holds a content. A pointer variable (or pointer in short) is basically the same as the other variables, but it stores a memory address.
The size of the address (and of course, the pointer) depends on the architecture of the computer. For example, in a 32-bit system, the size of the pointer is 4 bytes, while in a 64-bit system, the size of the pointer is 8 bytes.
Example
int *p; // declarationint x = 3;
p = &x; // assign the address of x to p
printf("%u %d\n", p, *p);// Output: 0xc7977ff934 3
*p = 5; // changes value of x to 5
void *p1; // can be used to store any address
2.3 Arrays
Declaration & Initialization:
int arr[5];int arr1[] = {1, 2, 3, 4, 5};printf("%d\n", arr1[2]);
A better pattern: single source of truth!
int ARRAY_SIZE = 5;int arr[ARRAY_SIZE];for (int i = 0; i < ARRAY_SIZE; i++) { arr[i] = i;}
2.4 Strings
In C language, strings are stored in an array of characters. The end of the string is
marked by a special character, the null character \0
.
char str[] = "Hello, World!";char str1[6] = {'H', 'e', 'l', 'l', 'o', '\0'};char str2[6] = "Hello";
Here are some commonly-used string functions defined in the string.h
library:
size_t strlen(const char * str)
: Returns the length of the string (not including the null terminator).char * strcpy ( char * destination, const char * source )
: Copies the C string pointed by source into the array pointed by destination, including the terminating null character (and stopping at that point).char * strncpy ( char * destination, const char * source, size_t num )
: Copies the first num characters of source to destination. If the end of the source C string (which is signaled by a null-character) is found before num characters have been copied, destination is padded with zeros until a total of num characters have been written to it.int strcmp ( const char * str1, const char * str2 );
: Compares the C stringstr1
to the C stringstr2
, return 0 if str1 and str2 are identical.int strncmp ( const char * str1, const char * str2, size_t num )
: Compares up to num characters of the C stringstr1
to those of the C stringstr2
.char * strcat ( char * destination, const char * source )
: Appends a copy of the source string to the destination string. The terminating null character in destination is overwritten by the first character of source, and a null-character is included at the end of the new string formed by the concatenation of both in destination.char * strncat ( char * destination, const char * source, size_t num )
: Appends the first num characters of source to destination, plus a terminating null-character.
3 Floating Point
Floating-point arithmetic (FP) is arithmetic on subsets of real numbers formed by a significand (a signed sequence of a fixed number of digits in some base) multiplied by an integer power of that base.
3.1 IEEE 754 Floating-Point Representation
The IEEE floating-point standard represents a number in a form .
- Sign determines whether the number is negative () or positive ().